用java语言编写程序,创建并运行3个线程:第一个线程打印100次字母a,第二个线程打印100次字母b,第三个线程打印1~100的整数。
时间: 2024-02-18 18:03:18 浏览: 28
下面是一个使用Java实现的例子:
```java
public class MyThread implements Runnable {
private char c;
private int start;
private int end;
public MyThread(char c, int start, int end) {
this.c = c;
this.start = start;
this.end = end;
}
public void run() {
for (int i = start; i <= end; i++) {
if (c == 'a') {
System.out.print('a');
} else if (c == 'b') {
System.out.print('b');
} else {
System.out.print(i + " ");
}
}
}
}
public class Main {
public static void main(String[] args) {
Thread threadA = new Thread(new MyThread('a', 1, 100));
Thread threadB = new Thread(new MyThread('b', 1, 100));
Thread threadC = new Thread(new MyThread('c', 1, 100));
threadA.start();
threadB.start();
threadC.start();
try {
threadA.join();
threadB.join();
threadC.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("\nDone");
}
}
```
在这个例子中,我们定义了一个MyThread类实现了Runnable接口,其中的构造函数接受三个参数:打印的字符c、起始值start和结束值end。在run方法中,我们使用for循环打印相应的字符或整数。然后,我们创建了三个线程,分别调用MyThread类的构造函数。最后,我们使用join方法等待三个线程全部执行完毕,并输出一个'Done'提示。这样,我们就成功地创建并运行了三个线程。
相关推荐
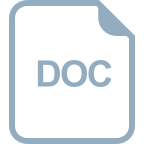
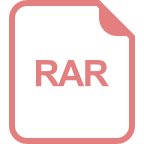
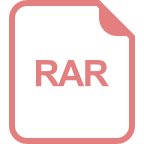

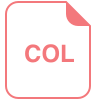
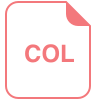











