stm32f031 flash读写代码
时间: 2023-08-29 11:12:36 浏览: 177
以下是一个简单的示例代码,用于在STM32F031微控制器上进行Flash读写操作:
```c
#include "stm32f0xx.h"
#define ADDR_FLASH_PAGE_127 ((uint32_t)0x0803F800) /* Flash 127 Page Address */
void flash_erase_page(uint32_t page_address)
{
FLASH_Unlock(); /* Unlock the Flash to enable the flash control register access */
/* Clear pending flags (if any) */
FLASH_ClearFlag(FLASH_FLAG_EOP | FLASH_FLAG_PGERR | FLASH_FLAG_WRPERR);
/* Erase the Flash page */
FLASH_ErasePage(page_address);
FLASH_Lock(); /* Lock the Flash to disable the flash control register access */
}
void flash_write_word(uint32_t address, uint32_t data)
{
FLASH_Unlock(); /* Unlock the Flash to enable the flash control register access */
/* Clear pending flags (if any) */
FLASH_ClearFlag(FLASH_FLAG_EOP | FLASH_FLAG_PGERR | FLASH_FLAG_WRPERR);
/* Write the word data into the specified address */
FLASH_ProgramWord(address, data);
FLASH_Lock(); /* Lock the Flash to disable the flash control register access */
}
uint32_t flash_read_word(uint32_t address)
{
return (*(__IO uint32_t*) address);
}
int main(void)
{
uint32_t data = 0x12345678;
uint32_t read_data;
flash_erase_page(ADDR_FLASH_PAGE_127); /* Erase the flash page */
flash_write_word(ADDR_FLASH_PAGE_127, data); /* Write data to flash */
read_data = flash_read_word(ADDR_FLASH_PAGE_127); /* Read data from flash */
return 0;
}
```
需要注意的是,Flash写入操作会擦除整个Flash页面,因此在写入之前需要先擦除页面。Flash读取操作可以直接从指定地址读取数据。同时,需要在进行Flash操作之前解锁Flash,操作完成之后再锁定Flash以保护Flash数据的完整性。
阅读全文
相关推荐
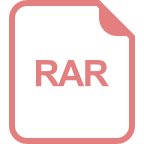
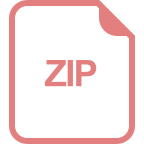
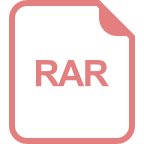
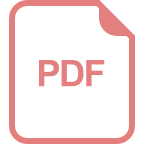
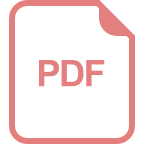








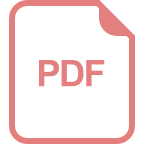
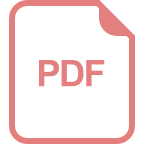
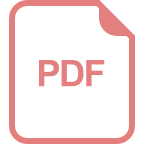