如何生成反走样(即抗锯齿)的直线? 请尝试编写代码。
时间: 2024-05-03 15:21:42 浏览: 78
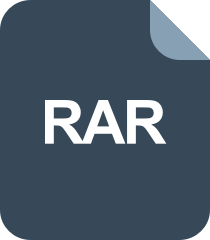
直线反走样算法

反走样直线是通过在直线边缘周围绘制多个像素来模拟平滑的线条的技术。以下是一种实现方法:
1.确定直线的起点和终点。
2.计算直线的斜率。
3.对于直线上的每个像素,计算该像素到直线的距离。这可以通过将像素坐标代入直线方程中来实现。
4.根据距离计算像素的权重。距离越小,像素的权重越高。
5.将像素的颜色乘以其权重,并将结果添加到输出像素的颜色中。
以下是一个示例代码,使用Python语言实现反走样直线绘制:
```
import numpy as np
import matplotlib.pyplot as plt
def antialiased_line(x0, y0, x1, y1):
"""Draw an antialiased line from (x0, y0) to (x1, y1)."""
# Determine the slope of the line
dx = abs(x1 - x0)
dy = abs(y1 - y0)
if x0 < x1:
sx = 1
else:
sx = -1
if y0 < y1:
sy = 1
else:
sy = -1
err = dx - dy
# Create a blank image to draw on
img = np.zeros((dy+1, dx+1))
# Loop over each pixel on the line
while True:
# Set the pixel color based on the distance from the line
x = x0 - max(x0-x1, 0)
y = y0 - max(y0-y1, 0)
d = abs(err)/dx+dy
w = 1-d
img[y,x] += w
img[y,x+sx] += d-w
img[y+sy,x] += d-w
img[y+sy,x+sx] += w-d
# Move to the next pixel
if x0 == x1 and y0 == y1:
break
e2 = 2*err
if e2 > -dy:
err -= dy
x0 += sx
if e2 < dx:
err += dx
y0 += sy
# Normalize the image
img /= img.max()
# Return the image
return img
# Draw an example line
x0, y0 = 20, 10
x1, y1 = 60, 50
img = antialiased_line(x0, y0, x1, y1)
# Display the image
plt.imshow(img, cmap='gray', interpolation='nearest')
plt.show()
```
该代码使用numpy和matplotlib库来生成图像。输出是一个灰度图像,其中较亮的像素代表直线的中心,较暗的像素代表直线的边缘。
阅读全文
相关推荐
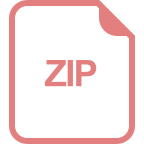

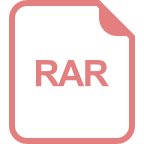

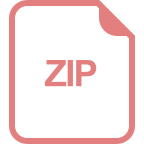
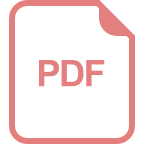
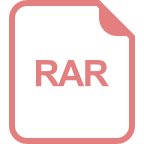
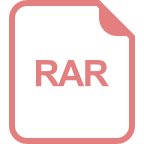
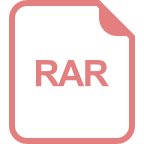
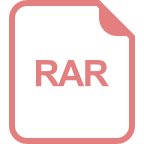
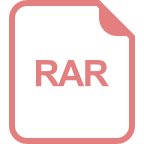





