有一个售货柜员机,内有多种商品供人购买(商品种类不超过100个),每种商品有 名称、数量、价等信息。c语言编程完成n种商品信息的输入输出
时间: 2024-02-23 11:58:36 浏览: 26
好的,我可以为您提供一个简单的C语言程序来实现这个功能。首先,我们需要定义一个结构体来表示每种商品的信息,如下所示:
```c
typedef struct {
char name[50]; // 商品名称
int quantity; // 商品数量
float price; // 商品价格
} Product;
```
然后,我们可以在程序中定义一个数组来存储所有商品的信息,如下所示:
```c
Product products[100];
```
接下来,我们可以编写一个函数来输入每种商品的信息,并将其存储到数组中,如下所示:
```c
void inputProducts(int n) {
for (int i = 0; i < n; i++) {
printf("请输入第%d种商品的名称、数量和价格:\n", i + 1);
scanf("%s %d %f", products[i].name, &products[i].quantity, &products[i].price);
}
}
```
最后,我们可以编写一个函数来输出所有商品的信息,如下所示:
```c
void outputProducts(int n) {
printf("所有商品的信息如下:\n");
for (int i = 0; i < n; i++) {
printf("%d. %s 数量:%d 价格:%.2f元\n", i + 1, products[i].name, products[i].quantity, products[i].price);
}
}
```
完整的程序如下所示:
```c
#include <stdio.h>
typedef struct {
char name[50];
int quantity;
float price;
} Product;
Product products[100];
void inputProducts(int n) {
for (int i = 0; i < n; i++) {
printf("请输入第%d种商品的名称、数量和价格:\n", i + 1);
scanf("%s %d %f", products[i].name, &products[i].quantity, &products[i].price);
}
}
void outputProducts(int n) {
printf("所有商品的信息如下:\n");
for (int i = 0; i < n; i++) {
printf("%d. %s 数量:%d 价格:%.2f元\n", i + 1, products[i].name, products[i].quantity, products[i].price);
}
}
int main() {
int n;
printf("请输入商品的种类数:\n");
scanf("%d", &n);
inputProducts(n);
outputProducts(n);
return 0;
}
```
当然,这只是一个简单的实现,您可以根据自己的需求进行修改和扩展。
相关推荐









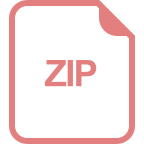
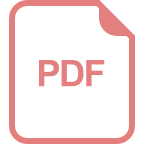
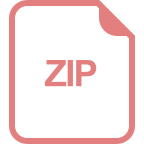
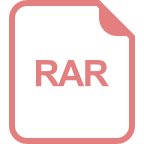
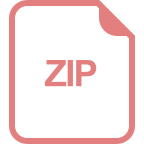
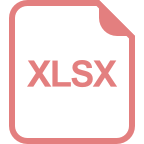