m = lambda x: torch.maximum(torch.abs(x)-torch.ones_like(x),torch.zeros_like(x))
时间: 2023-10-20 20:03:48 浏览: 140
这是一个使用PyTorch实现的函数,它的作用是将输入的x中的每个元素取绝对值后减去1,如果结果小于0,则将其设为0,否则保留原值。这个函数可以用于实现L1正则化。具体来说,如果我们在训练神经网络时希望对网络的权重进行L1正则化,就可以将每个权重矩阵作为输入传给这个函数,然后将函数的输出作为正则化项加入损失函数中,从而鼓励权重矩阵中的大部分元素都接近于0,从而实现参数的稀疏化。
相关问题
s = 256 # 2x min stride m.inplace = self.inplace forward = lambda x: self.forward(x)[0] if isinstance(m, Segment) else self.forward(x) m.stride = torch.tensor([s / x.shape[-2] for x in forward(torch.zeros(1, ch, s, s))]) # forward check_anchor_order(m) m.anchors /= m.stride.view(-1, 1, 1) self.stride = m.stride self._initialize_biases() # only run once
这段代码看起来像是在进行目标检测模型的初始化过程,其中包括计算输入图像的 stride,调整锚框的大小以适应不同尺度的特征图,以及初始化偏置参数等。具体来说:
- 代码开头的 s = 256 表示输入图像的大小为 256x256。
- 接下来的 forward 函数是为了通过模型前向传播得到特征图的大小,以便计算 stride。其中 isinstance(m, Segment) 是为了检查模型是否为分割模型,因为分割模型需要将输出的大小与输入大小一致。
- 然后通过计算 s / x.shape[-2] 得到 stride,其中 x.shape[-2] 是特征图的高度或宽度,取其中较小值是为了保证特征图不会超出输入图像的大小。
- check_anchor_order 函数是为了检查锚框的大小是否符合规范。
- 最后通过将锚框大小除以 stride,得到调整后的锚框大小,以适应不同尺度的特征图。
值得注意的是,这段代码中还有一些其他函数和参数,可能需要结合具体模型来理解其含义。
下面pytorch代码转化为paddlepaddle2.2.2代码: import torch import torch.distributions as tdist def random_noise_levels(): """Generates random noise levels from a log-log linear distribution.""" log_min_shot_noise = np.log(0.0001) log_max_shot_noise = np.log(0.012) log_shot_noise = torch.FloatTensor(1).uniform_(log_min_shot_noise, log_max_shot_noise) shot_noise = torch.exp(log_shot_noise) line = lambda x: 2.18 * x + 1.20 n = tdist.Normal(loc=torch.tensor([0.0]), scale=torch.tensor([0.26])) log_read_noise = line(log_shot_noise) + n.sample() read_noise = torch.exp(log_read_noise) return shot_noise, read_noise def add_noise(image, shot_noise=0.01, read_noise=0.0005): """Adds random shot (proportional to image) and read (independent) noise.""" image = image.permute(1, 2, 0) # Permute the image tensor to HxWxC format from CxHxW format variance = image * shot_noise + read_noise n = tdist.Normal(loc=torch.zeros_like(variance), scale=torch.sqrt(variance)) noise = n.sample() out = image + noise out = out.permute(2, 0, 1) # Re-Permute the tensor back to CxHxW format return out
以下是将该代码转化为PaddlePaddle2.2.2的代码:
```
import paddle
import paddle.distribution as pdist
import numpy as np
def random_noise_levels():
"""Generates random noise levels from a log-log linear distribution."""
log_min_shot_noise = np.log(0.0001)
log_max_shot_noise = np.log(0.012)
log_shot_noise = paddle.uniform(shape=[1], min=log_min_shot_noise, max=log_max_shot_noise)
shot_noise = paddle.exp(log_shot_noise)
line = lambda x: 2.18 * x + 1.20
n = pdist.Normal(loc=paddle.zeros([1]), scale=paddle.ones([1]) * 0.26)
log_read_noise = line(log_shot_noise) + n.sample()
read_noise = paddle.exp(log_read_noise)
return shot_noise, read_noise
def add_noise(image, shot_noise=0.01, read_noise=0.0005):
"""Adds random shot (proportional to image) and read (independent) noise."""
image = image.permute([1, 2, 0]) # Permute the image tensor to HxWxC format from CxHxW format
variance = image * shot_noise + read_noise
n = pdist.Normal(loc=paddle.zeros_like(variance), scale=paddle.sqrt(variance))
noise = n.sample()
out = image + noise
out = out.permute([2, 0, 1]) # Re-Permute the tensor back to CxHxW format
return out
```
请注意,PaddlePaddle的分布API(`paddle.distribution`)与PyTorch的分布API(`torch.distributions`)略有不同,需要相应地更改代码。此外,PaddlePaddle的张量API(如`paddle.exp`)与PyTorch的张量API(如`torch.exp`)也略有不同,需要相应地更改代码。
阅读全文
相关推荐

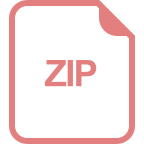
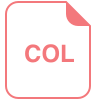
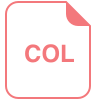
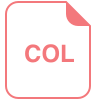
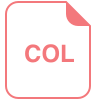
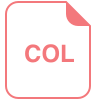
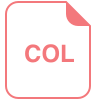
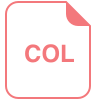
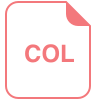
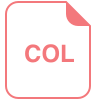
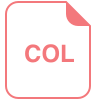
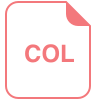