将 h5 文件转换为 tflite 格式
时间: 2023-12-17 11:03:25 浏览: 146
要将H5文件转换为TFLite格式,您可以按照以下步骤进行操作:
1. 安装TensorFlow和TensorFlow Lite的Python库:
```
pip install tensorflow tensorflow-lite
```
2. 导入TensorFlow库并加载H5模型:
```python
import tensorflow as tf
# 加载H5模型
model = tf.keras.models.load_model('path/to/your_model.h5')
```
3. 将模型转换为TFLite格式:
```python
# 转换为TFLite格式
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
```
4. 将TFLite模型保存到文件:
```python
with open('path/to/your_model.tflite', 'wb') as f:
f.write(tflite_model)
```
现在,您已经成功将H5模型转换为TFLite格式,并将其保存为一个.tflite文件。请注意,转换过程中可能会有一些限制和约束,例如某些高级功能可能不支持或转换后的模型大小可能会有所增加。因此,在转换前请确保您的模型和应用程序的需求是兼容的。
相关问题
怎么把h5文件变成tflite格式
要将 h5 文件转换为 tflite 格式,您可以按照以下步骤进行操作:
1. 安装 TensorFlow:确保您已经安装了 TensorFlow 库,可以使用以下命令安装:
```
pip install tensorflow
```
2. 加载模型:使用 TensorFlow 加载 h5 模型文件并还原模型的结构和权重。例如,可以使用以下代码加载模型:
```python
import tensorflow as tf
# 加载 h5 模型
model = tf.keras.models.load_model('model.h5')
```
3. 量化模型:tflite 格式需要对模型进行量化,即将模型参数转换为低精度表示。可以使用 TensorFlow 提供的量化工具来实现。以下是一个示例代码:
```python
# 创建量化转换器
converter = tf.lite.TFLiteConverter.from_keras_model(model)
# 设置优化选项(可选)
converter.optimizations = [tf.lite.Optimize.DEFAULT]
# 进行量化转换
tflite_model = converter.convert()
```
4. 保存为 tflite 文件:将量化后的模型保存为 tflite 文件,可以使用以下代码:
```python
# 保存为 tflite 文件
with open('model.tflite', 'wb') as f:
f.write(tflite_model)
```
现在,您就可以在 TensorFlow Lite 环境中使用生成的 tflite 模型文件进行推理了。请注意,转换为 tflite 格式可能会对模型的精度和性能产生一定影响,可以根据具体需求进行优化设置和调整。
用python写一个h5格式转为tflite格式的代码
以下是一个将H5模型转换为TFLite模型的Python代码示例:
```
import tensorflow as tf
# 加载H5模型
model = tf.keras.models.load_model('model.h5')
# 转换模型为TFLite模型
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
# 保存TFLite模型
with open('model.tflite', 'wb') as f:
f.write(tflite_model)
```
在代码中,首先使用`tf.keras.models.load_model`加载H5模型。然后,使用`tf.lite.TFLiteConverter.from_keras_model`创建一个转换器对象,将加载的H5模型转换为TFLite模型。最后,将TFLite模型保存到文件中,使用`with open`语句打开文件,将模型写入文件中。
阅读全文
相关推荐
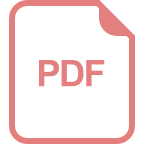
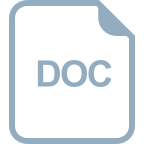
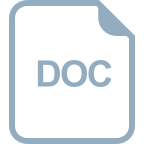
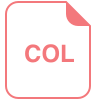






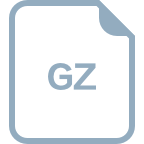
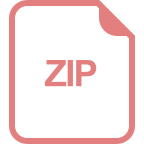



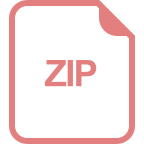