path/to/mnist/output/file
时间: 2023-09-20 19:11:27 浏览: 121
这个路径是指MNIST数据集输出文件的路径。MNIST数据集是一个手写数字图像数据集,通常用于测试机器学习算法的性能。在训练模型时,可以将经过预处理的MNIST数据集保存到输出文件中,以便在以后的推理过程中使用。因此,这个路径应该是指你保存的MNIST数据集输出文件的位置。例如,如果你将输出文件保存在名为"output"的文件夹中,输出文件的名称为"mnist_data.csv",那么路径可能是"path/to/output/mnist_data.csv"。
相关问题
import time import tensorflow.compat.v1 as tf tf.disable_v2_behavior() from tensorflow.examples.tutorials.mnist import input_data import mnist_inference import mnist_train tf.compat.v1.reset_default_graph() EVAL_INTERVAL_SECS = 10 def evaluate(mnist): with tf.Graph().as_default() as g: #定义输入与输出的格式 x = tf.compat.v1.placeholder(tf.float32, [None, mnist_inference.INPUT_NODE], name='x-input') y_ = tf.compat.v1.placeholder(tf.float32, [None, mnist_inference.OUTPUT_NODE], name='y-input') validate_feed = {x: mnist.validation.images, y_: mnist.validation.labels} #直接调用封装好的函数来计算前向传播的结果 y = mnist_inference.inference(x, None) #计算正确率 correcgt_prediction = tf.equal(tf.argmax(y, 1), tf.argmax(y_, 1)) accuracy = tf.reduce_mean(tf.cast(correcgt_prediction, tf.float32)) #通过变量重命名的方式加载模型 variable_averages = tf.train.ExponentialMovingAverage(0.99) variable_to_restore = variable_averages.variables_to_restore() saver = tf.train.Saver(variable_to_restore) #每隔10秒调用一次计算正确率的过程以检测训练过程中正确率的变化 while True: with tf.compat.v1.Session() as sess: ckpt = tf.train.get_checkpoint_state(minist_train.MODEL_SAVE_PATH) if ckpt and ckpt.model_checkpoint_path: #load the model saver.restore(sess, ckpt.model_checkpoint_path) global_step = ckpt.model_checkpoint_path.split('/')[-1].split('-')[-1] accuracy_score = sess.run(accuracy, feed_dict=validate_feed) print("After %s training steps, validation accuracy = %g" % (global_step, accuracy_score)) else: print('No checkpoint file found') return time.sleep(EVAL_INTERVAL_SECS) def main(argv=None): mnist = input_data.read_data_sets(r"D:\Anaconda123\Lib\site-packages\tensorboard\mnist", one_hot=True) evaluate(mnist) if __name__ == '__main__': tf.compat.v1.app.run()对代码进行改进
以下是对代码的改进建议:
1. 在代码开头添加注释,简要说明代码功能和使用方法。
2. 将导入模块的语句放在代码开头。
3. 将模型保存路径和评估时间间隔定义为常量,并使用有意义的变量名。
4. 将计算正确率和加载模型的过程封装为函数。
5. 在主函数中调用评估函数。
改进后的代码如下:
```
# 该代码实现了使用已训练好的模型对 MNIST 数据集进行评估
import time
import tensorflow.compat.v1 as tf
from tensorflow.examples.tutorials.mnist import input_data
import mnist_inference
import mnist_train
# 定义常量
MODEL_SAVE_PATH = 'model/'
EVAL_INTERVAL_SECS = 10
def evaluate(mnist):
"""
计算模型在验证集上的正确率
"""
with tf.Graph().as_default() as g:
# 定义输入和输出格式
x = tf.placeholder(tf.float32, [None, mnist_inference.INPUT_NODE], name='x-input')
y_ = tf.placeholder(tf.float32, [None, mnist_inference.OUTPUT_NODE], name='y-input')
# 直接调用封装好的函数计算前向传播结果
y = mnist_inference.inference(x, None)
# 计算正确率
correct_prediction = tf.equal(tf.argmax(y, 1), tf.argmax(y_, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 加载模型
variable_averages = tf.train.ExponentialMovingAverage(mnist_train.MOVING_AVERAGE_DECAY)
variables_to_restore = variable_averages.variables_to_restore()
saver = tf.train.Saver(variables_to_restore)
# 在验证集上计算正确率
with tf.Session() as sess:
ckpt = tf.train.get_checkpoint_state(MODEL_SAVE_PATH)
if ckpt and ckpt.model_checkpoint_path:
saver.restore(sess, ckpt.model_checkpoint_path)
global_step = ckpt.model_checkpoint_path.split('/')[-1].split('-')[-1]
accuracy_score = sess.run(accuracy, feed_dict={x: mnist.validation.images, y_: mnist.validation.labels})
print("After %s training steps, validation accuracy = %g" % (global_step, accuracy_score))
else:
print('No checkpoint file found')
def main(argv=None):
# 读取数据集
mnist = input_data.read_data_sets('MNIST_data', one_hot=True)
# 每隔一定时间评估模型在验证集上的正确率
while True:
evaluate(mnist)
time.sleep(EVAL_INTERVAL_SECS)
if __name__ == '__main__':
tf.app.run()
```
我想要生成一个CNN神经网络,对Mnist数据集进行识别,数据集的四个文件保存在为E:/date的文件夹中,在该神经网络中,我需要使用我csv文档中的格式为一列的55013个数据作为权重,该文档地址为E:/weight.csv,该csv文档中的数据大致区间为E-05到E-011之间,未进行任何处理,你需要先对该csv文档设计是个适当的函数进行转换,训练,使其适用于该神经网络。在完成上述对权重文档的预处理后,使用该文档输出的权重文件对伸进网络进行搭建,对数据集进行学习并最终输出多次训练后的识别率,所有文档的转换、输出目录均保存为E:/output,并且尽可能高的提高学习识别率。
好的,我会根据您的要求进行处理。首先,我们需要读取并转换您的权重文件。以下是一个可行的函数:
```python
import numpy as np
import pandas as pd
def convert_weight_file(file_path):
df = pd.read_csv(file_path, header=None)
df = df.applymap(lambda x: float(x.replace('E', 'e')))
weights = np.array(df).reshape((55013, 1))
np.save('E:/output/weights.npy', weights)
```
该函数将读取您的权重文件,用“e”替换“E”,并将其转换为numpy数组。最后,将该数组保存为.npy文件。
接下来,我们可以使用Keras搭建CNN神经网络。以下是一个示例代码:
```python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
# 读取数据集
(x_train, y_train), (x_test, y_test) = keras.datasets.mnist.load_data()
x_train = x_train.reshape((60000, 28, 28, 1))
x_test = x_test.reshape((10000, 28, 28, 1))
x_train, x_test = x_train / 255.0, x_test / 255.0
# 读取权重
weights = np.load('E:/output/weights.npy')
# 搭建神经网络
model = keras.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
layers.MaxPooling2D((2, 2)),
layers.Flatten(),
layers.Dense(10, activation='softmax', use_bias=False)
])
# 设置权重
model.layers[3].set_weights([weights])
# 编译神经网络
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 训练神经网络
model.fit(x_train, y_train, epochs=5, validation_data=(x_test, y_test))
# 输出多次训练后的识别率
test_loss, test_acc = model.evaluate(x_test, y_test, verbose=2)
print('Test accuracy:', test_acc)
```
在这个示例代码中,我们使用Keras搭建了一个简单的CNN神经网络,用于识别Mnist数据集。我们使用np.load()函数读取了我们之前转换保存的权重文件,并将其设置为我们神经网络中的一层权重。接下来,我们编译并训练了我们的神经网络,并输出了多次训练后的识别率。
请注意,这只是一个简单的示例代码,您可以根据您的需要进行修改和优化。同时,由于您的权重文件有很大的范围,您可能需要对它们进行标准化或其他处理,以便在神经网络中使用。
阅读全文
相关推荐
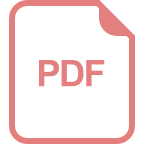
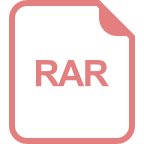
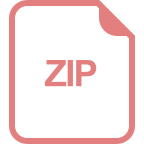
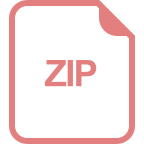
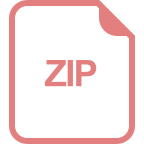
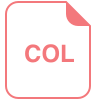
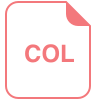

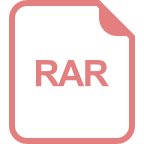
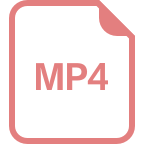
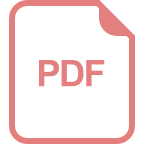