WPF 两层嵌套ListBox如何响应对应层的操作
时间: 2024-01-12 19:02:53 浏览: 30
在WPF中实现两层嵌套ListBox响应对应层的操作,可以通过以下两种方式实现:
1. 使用命令绑定:在每一层的数据模板中分别绑定对应的命令,使用CommandParameter传递需要操作的数据对象。在ViewModel中实现命令的具体逻辑,根据传递的参数执行对应操作。
示例XAML代码如下:
```xaml
<ListBox ItemsSource="{Binding ParentItems}">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel>
<TextBlock Text="{Binding Name}" />
<ListBox ItemsSource="{Binding ChildItems}">
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding Name}">
<i:Interaction.Triggers>
<i:EventTrigger EventName="MouseDoubleClick">
<i:InvokeCommandAction Command="{Binding DataContext.ChildItemCommand, RelativeSource={RelativeSource AncestorType={x:Type ListBox}}}" CommandParameter="{Binding}" />
</i:EventTrigger>
</i:Interaction.Triggers>
</TextBlock>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
```
示例ViewModel代码如下:
```csharp
public class MyViewModel
{
public ICommand ParentItemCommand { get; set; }
public ICommand ChildItemCommand { get; set; }
public MyViewModel()
{
ParentItemCommand = new RelayCommand<ParentItem>(ExecuteParentItemCommand);
ChildItemCommand = new RelayCommand<ChildItem>(ExecuteChildItemCommand);
}
private void ExecuteParentItemCommand(ParentItem item)
{
// 处理父级ListBox的操作
}
private void ExecuteChildItemCommand(ChildItem item)
{
// 处理子级ListBox的操作
}
}
```
2. 使用事件:在每一层的ListBox中添加对应的事件处理程序,根据事件参数中的数据对象执行对应操作。需要注意的是,由于子级ListBox会触发父级ListBox的事件,因此需要在事件处理程序中判断事件源是哪一层的ListBox。
示例XAML代码如下:
```xaml
<ListBox ItemsSource="{Binding ParentItems}" MouseDoubleClick="ParentListBox_MouseDoubleClick">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel>
<TextBlock Text="{Binding Name}" />
<ListBox ItemsSource="{Binding ChildItems}" MouseDoubleClick="ChildListBox_MouseDoubleClick">
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding Name}" />
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
```
示例代码behind代码如下:
```csharp
private void ParentListBox_MouseDoubleClick(object sender, MouseButtonEventArgs e)
{
var parentListBox = sender as ListBox;
if (parentListBox != null && parentListBox.SelectedItem != null)
{
var item = parentListBox.SelectedItem as ParentItem;
// 处理父级ListBox的操作
}
}
private void ChildListBox_MouseDoubleClick(object sender, MouseButtonEventArgs e)
{
var childListBox = sender as ListBox;
if (childListBox != null && childListBox.SelectedItem != null)
{
var item = childListBox.SelectedItem as ChildItem;
// 处理子级ListBox的操作
}
}
```
以上两种方式都可以实现两层嵌套ListBox响应对应层的操作,具体选择哪种方式取决于您的具体需求和代码习惯。
相关推荐
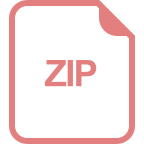














