js获取数组树结构中某个节点及其所有的父节点
时间: 2023-09-02 20:09:31 浏览: 120
可以使用递归的方式来实现获取数组树结构中某个节点及其所有的父节点。
具体实现可以参考下面的代码:
```javascript
function findNodeAndParents(tree, nodeId) {
let result = [];
for (let i = 0; i < tree.length; i++) {
let node = tree[i];
if (node.id === nodeId) {
result.push(node);
return result;
} else if (node.children) {
let childResult = findNodeAndParents(node.children, nodeId);
if (childResult.length) {
result.push(node);
result = result.concat(childResult);
return result;
}
}
}
return result;
}
```
其中,`tree` 表示要查找的数组树结构,`nodeId` 表示要查找的节点的 ID。
函数返回一个数组,包含要查找的节点及其所有的父节点。
使用示例:
```javascript
let tree = [
{
id: 1,
name: '节点1',
children: [
{
id: 2,
name: '节点2',
children: [
{
id: 3,
name: '节点3'
}
]
},
{
id: 4,
name: '节点4'
}
]
},
{
id: 5,
name: '节点5',
children: [
{
id: 6,
name: '节点6',
children: [
{
id: 7,
name: '节点7'
}
]
},
{
id: 8,
name: '节点8'
}
]
}
];
let result = findNodeAndParents(tree, 3);
console.log(result); // [{id: 1, name: "节点1"}, {id: 2, name: "节点2"}, {id: 3, name: "节点3"}]
```
阅读全文
相关推荐
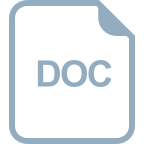




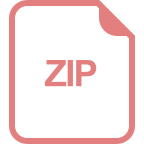
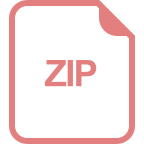
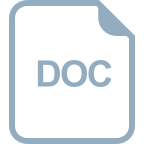
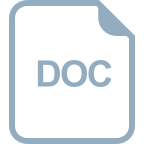
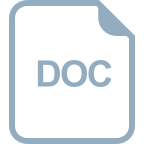






