js获取数组树结构中某个节点及其所有的父节点,该节点包含id,name,children等元素,并以树结构形式返回
时间: 2023-12-06 15:39:44 浏览: 33
可以使用递归方式来实现获取数组树结构中某个节点及其所有的父节点:
```javascript
function findNodeById(tree, id, path = []) {
for (let i = 0; i < tree.length; i++) {
const node = tree[i];
if (node.id === id) {
// 找到目标节点,返回路径
return [...path, node];
} else if (node.children) {
// 递归遍历子节点
const result = findNodeById(node.children, id, [...path, node]);
if (result) {
// 找到目标节点,返回路径
return result;
}
}
}
// 没有找到目标节点
return null;
}
```
这个函数接受三个参数:
- `tree`:树结构数组
- `id`:要查找的节点的 ID
- `path`:当前路径数组,用于记录最终路径
如果找到了目标节点,就返回路径数组;如果没有找到,就返回 `null`。
使用示例:
```javascript
const tree = [
{
id: 1,
name: '节点1',
children: [
{
id: 2,
name: '节点2',
children: [
{
id: 3,
name: '节点3',
},
{
id: 4,
name: '节点4',
},
],
},
{
id: 5,
name: '节点5',
},
],
},
{
id: 6,
name: '节点6',
children: [
{
id: 7,
name: '节点7',
children: [
{
id: 8,
name: '节点8',
},
{
id: 9,
name: '节点9',
},
],
},
],
},
];
const path = findNodeById(tree, 4);
console.log(path); // 输出:[ { id: 1, name: '节点1', ... }, { id: 2, name: '节点2', ... }, { id: 4, name: '节点4', ... } ]
```
这个示例中,我们调用 `findNodeById` 函数,查找 ID 为 4 的节点,并返回其路径数组。最终输出结果为 `[ { id: 1, name: '节点1', ... }, { id: 2, name: '节点2', ... }, { id: 4, name: '节点4', ... } ]`,表示从根节点开始,依次经过节点 1、节点 2 和节点 4,才能到达目标节点。
相关推荐
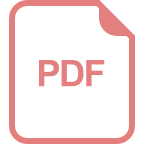
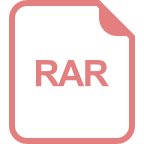
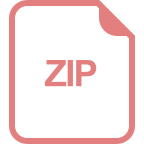
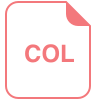













