js获取树结构某个节点及其所有父节点组成的树结构
时间: 2023-06-26 09:05:26 浏览: 47
可以使用递归来获取某个节点及其所有父节点组成的树结构。假设你的树结构是一个类似下面的对象:
```javascript
const treeData = {
id: 1,
name: 'Node 1',
children: [
{
id: 2,
name: 'Node 2',
children: [
{
id: 3,
name: 'Node 3',
children: []
},
{
id: 4,
name: 'Node 4',
children: []
}
]
},
{
id: 5,
name: 'Node 5',
children: []
}
]
};
```
你可以使用下面的代码来获取指定节点及其所有父节点组成的树结构:
```javascript
function getNodeAndParents(nodeId, tree) {
if (tree.id === nodeId) {
return tree;
}
for (let child of tree.children) {
const node = getNodeAndParents(nodeId, child);
if (node) {
return {
id: tree.id,
name: tree.name,
children: [node]
};
}
}
return null;
}
```
使用方法如下:
```javascript
const node = getNodeAndParents(4, treeData);
console.log(node);
```
输出结果如下:
```javascript
{
id: 1,
name: 'Node 1',
children: [
{
id: 2,
name: 'Node 2',
children: [
{
id: 4,
name: 'Node 4',
children: []
}
]
}
]
}
```
这个函数会返回一个包含指定节点及其所有父节点的树结构。如果指定的节点不存在于树中,则返回 null。
相关推荐
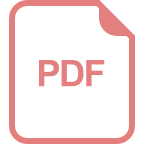
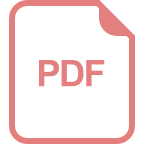
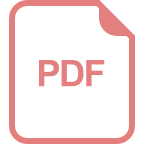
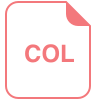
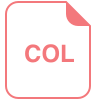
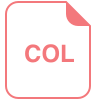
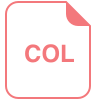










