1.定义一个名为Rectangle的类来表示矩形,这个类包括: (1)两个名为width和height的double类型数据域,它们分别表示矩形的宽和高。width和height的默认值都为1。 (2)一个用于创建默认矩形的无参构造方法。 (3)一个创建指定width和height值的矩形的构造方法。 (4)一个名为getArea()的方法,返回该矩形的面积。 (5)一个名为getPerimeter()的方法,返回周长。 2.定义一个MyRectangle类,它不但具有Rectangle类所有的功能,它还具有如下功能: (1)重写toString方法。假设当前对象的长和高是3和2,那么toString方法返回“width: 3, height:2”。 (2)重写equals方法。当两个MyRectangle实例的面积相等时,认为两个对象是相等的。 3.实现Comparable接口,使得MyRectangle对象可以按照面积大小排序: (1)实现Comparable接口,面积作为比较依据。 (2)创建MyRectangle类型的数组,每个元素都是MyRectangle对象。 (3)通过Arrays.sort方法对MyRectangle对象数组排序,并输出排序后的结果。
时间: 2024-03-24 20:37:21 浏览: 59
好的,下面是一个可能的解法:
Rectangle类:
```java
public class Rectangle {
private double width;
private double height;
public Rectangle() {
this.width = 1;
this.height = 1;
}
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getArea() {
return width * height;
}
public double getPerimeter() {
return 2 * (width + height);
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
}
```
MyRectangle类:
```java
public class MyRectangle extends Rectangle implements Comparable<MyRectangle> {
public MyRectangle() {
super();
}
public MyRectangle(double width, double height) {
super(width, height);
}
@Override
public String toString() {
return "width: " + getWidth() + ", height: " + getHeight();
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (!(obj instanceof MyRectangle)) {
return false;
}
MyRectangle other = (MyRectangle) obj;
return Double.compare(getArea(), other.getArea()) == 0;
}
@Override
public int compareTo(MyRectangle other) {
return Double.compare(getArea(), other.getArea());
}
}
```
测试代码:
```java
import java.util.Arrays;
public class Test {
public static void main(String[] args) {
MyRectangle[] rectangles = new MyRectangle[5];
rectangles[0] = new MyRectangle(3, 5);
rectangles[1] = new MyRectangle(2, 4);
rectangles[2] = new MyRectangle(1, 6);
rectangles[3] = new MyRectangle(4, 2);
rectangles[4] = new MyRectangle(5, 3);
Arrays.sort(rectangles);
for (MyRectangle rectangle : rectangles) {
System.out.println(rectangle.getArea());
}
}
}
```
输出结果:
```
6.0
8.0
10.0
15.0
20.0
```
说明矩形已经按照面积大小排序。
阅读全文
相关推荐
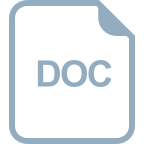
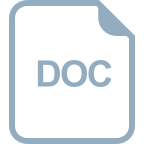
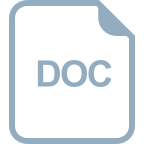










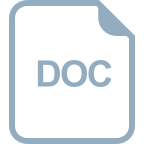
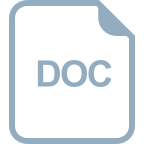
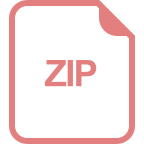
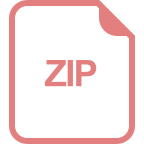
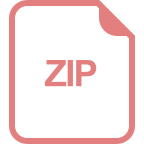