1.写一个名为rectangle的类表示矩形。其属性包括宽width、高height和颜色color,width和height都是double型的,而color则是string类型的。要求该类具有:
时间: 2023-05-31 19:20:16 浏览: 140
### 回答1:
这是一个名为rectangle的类表示矩形。它的属性包括宽度width、高度height和颜色color,其中width和height都是double类型,而color则是string类型。要求该类具有:
### 回答2:
Rectangle类是一个用于表示矩形的类,其主要属性包括宽度、高度和颜色。这个类应该可以根据提供的宽度、高度和颜色创建一个矩形实例。
首先,我们来看看如何定义这些属性。在C++中,我们可以使用类中的数据成员来定义宽、高和颜色:
```
class Rectangle {
private:
double width;
double height;
string color;
};
```
下一步是如何设置和获取这些属性的值。为了能够设置和获取这些属性,我们可以使用类中的成员函数:
```
class Rectangle {
private:
double width;
double height;
string color;
public:
void setWidth(double);
double getWidth();
void setHeight(double);
double getHeight();
void setColor(string);
string getColor();
};
void Rectangle::setWidth(double w) {
width = w;
}
double Rectangle::getWidth() {
return width;
}
void Rectangle::setHeight(double h) {
height = h;
}
double Rectangle::getHeight() {
return height;
}
void Rectangle::setColor(string c) {
color = c;
}
string Rectangle::getColor() {
return color;
}
```
现在我们已经定义了属性和函数来设置和获取它们,我们需要定义一个构造函数来创建实例。构造函数可以使用以下方式定义:
```
class Rectangle {
private:
double width;
double height;
string color;
public:
Rectangle(double w, double h, string c) {
width = w;
height = h;
color = c;
}
// set and get functions here...
};
```
现在我们就可以创建一个新的矩形实例:
```
Rectangle r(10.0, 5.0, "green");
```
最后,我们需要定义一些其他的函数来对矩形进行操作,比如计算它的面积。我们可以定义一个计算面积的函数:
```
class Rectangle {
private:
double width;
double height;
string color;
public:
Rectangle(double w, double h, string c) {
width = w;
height = h;
color = c;
}
// set and get functions here...
double getArea() {
return width * height;
}
};
```
现在我们已经定义了一个基本的Rectangle类,它具有宽度、高度、颜色和计算面积的方法。我们可以使用这个类来创建任意数量的矩形,并对它们进行操作。
### 回答3:
Rectangle类是用于表示矩形的一个类。它包括了宽(width)、高(height)和颜色(color)三个属性。
首先,我们需要定义一个类名为Rectangle。对于宽(width)和高(height)的属性,需要声明为double型的数据。颜色(color)则需要声明为string型的数据。
在定义好属性后,我们需要编写一些方法来使得这个类能够操作这些属性。首先,我们需要编写一个构造器,来完成对象的初始化。
在构造器中,我们可以通过传入参数的形式,来初始化这个矩形的属性。例如,我们可以设置一个如下的构造器:
public Rectangle(double width, double height, string color) {
this.width = width;
this.height = height;
this.color = color;
}
接下来,我们需要编写一些Getter和Setter方法来获取和设置属性。这些方法可以使得外部程序可以读取和修改对象的属性。
例如,我们可以编写一个获取宽度(width)的方法:
public double getWidth() {
return this.width;
}
接下来,我们可以编写一个设置宽度(width)的方法:
public void setWidth(double width) {
this.width = width;
}
同样,我们也可以编写获取高度(height)和设置高度(height)的方法。
接下来,我们可以编写一些针对矩形的操作。例如,我们可以编写一个计算面积的方法:
public double getArea() {
return this.width * this.height;
}
除此之外,我们还可以编写一些针对颜色(color)的操作。例如,我们可以编写一个获取颜色(color)的方法:
public string getColor() {
return this.color;
}
也可以编写一个设置颜色(color)的方法:
public void setColor(string color) {
this.color = color;
}
总之,Rectangle类是一个非常简单的类,它用于表示矩形对象,并且提供了一些针对矩形的操作。它的属性包括宽度(width)、高度(height)和颜色(color),并且提供了Getter和Setter方法来获取和设置这些属性。同时,在这个类中,我们也编写了一些依赖于这些属性的方法来实现一些针对矩形的操作。
阅读全文
相关推荐
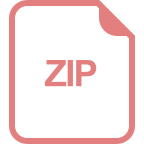
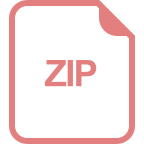











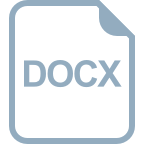
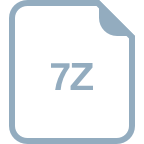