定义一个python类Rectangle,包含属性width和height,以及一个方法area用于计算矩形的面积
时间: 2024-10-17 07:12:25 浏览: 5
在Python中,你可以定义一个名为`Rectangle`的类来表示矩形,包含宽度(`width`)和高度(`height`)作为实例变量,并定义一个`area`方法来计算并返回矩形的面积。下面是一个简单的示例:
```python
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
"""
计算矩形的面积
"""
return self.width * self.height
# 创建一个矩形对象
my_rectangle = Rectangle(5, 4)
# 调用area方法
rectangle_area = my_rectangle.area()
print(f"The area of the rectangle is: {rectangle_area}")
```
在这个例子中,`__init__`方法是一个特殊的方法,当我们创建`Rectangle`类的新实例时,它会被自动调用。`area`方法则直接返回矩形的面积。
相关问题
编写一个Python类 Rectangle,具有属性 width 和 height,以及一个方法 area(),该方法计算矩形的面积。
在 Python 中,你可以创建一个名为 `Rectangle` 的类,它有两个属性 `width` 和 `height` 来表示矩形的宽度和高度,同时包含一个方法 `area()` 来计算矩形的面积。下面是这个类的一个简单实现:
```python
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
"""
计算并返回矩形的面积
"""
return self.width * self.height
```
在这个类中,`__init__` 方法是一个特殊的方法,当创建一个新的 `Rectangle` 对象时会自动调用,用来初始化 `width` 和 `height` 属性。`area` 方法则是直接根据矩形的宽和高相乘得到面积。
要使用这个类,你可以这样做:
```python
rectangle = Rectangle(5, 7)
print(rectangle.area()) # 输出 35
```
编写一个 Python 类 Rectangle ,具有属性 width 和 height ,以及一个方法 area (),该方法计算矩形的面积。
当然,我们可以创建一个名为 `Rectangle` 的 Python 类,用来表示矩形,并包含 `width` 和 `height` 属性,以及一个计算面积的方法 `area()`。以下是详细的实现:
```python
class Rectangle:
def __init__(self, width, height):
"""
初始化一个矩形,设置宽度和高度。
参数:
width -- 矩形的高度
"""
self.width = width
self.height = height
def area(self):
"""
计算并返回矩形的面积。
返回值:
返回矩形的面积,即 width 乘以 height.
"""
return self.width * self.height
# 示例用法
my_rectangle = Rectangle(5, 7)
print(my_rectangle.area()) # 输出 35
```
在这个类中,`__init__` 方法是一个特殊的方法,当创建一个新的 `Rectangle` 实例时会自动调用,用来初始化矩形的尺寸。`area()` 方法则是根据矩形的宽和高简单地计算面积。
阅读全文
相关推荐
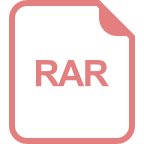
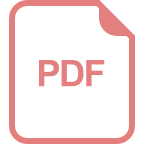
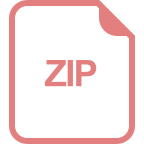













