yml如何按环境切换配置文件
时间: 2023-09-07 08:12:15 浏览: 62
在yml中按环境切换配置文件,可以通过Spring框架的Profile功能来实现。具体步骤如下:
1. 在yml中定义多个配置文件,每个配置文件对应不同的环境,如:
```
application-dev.yml #开发环境配置文件
application-prod.yml #生产环境配置文件
```
2. 在application.yml中指定当前环境使用的配置文件,如:
```
spring:
profiles:
active: dev #指定当前环境为开发环境
```
3. 在启动类上添加@Profile注解,指定当前启动的环境,如:
```
@SpringBootApplication
@Profile("dev") #指定当前启动的环境为开发环境
public class Application {
// ...
}
```
这样在启动应用程序时,就会根据指定的环境自动加载对应的配置文件,从而实现按环境切换配置文件的功能。
相关问题
springboot多环境yml配置
### 回答1:
Spring Boot支持多环境配置,可以通过在application.yml文件中定义不同的配置文件来实现。具体步骤如下:
1. 在src/main/resources目录下创建application.yml文件,用于存放公共配置。
2. 在同一目录下创建application-dev.yml、application-test.yml、application-prod.yml等文件,用于存放不同环境的配置。
3. 在application.yml文件中使用spring.profiles.active属性指定当前环境,如:
```
spring:
profiles:
active: dev
```
4. 在不同环境的配置文件中定义相应的属性,如:
```
server:
port: 808
spring:
datasource:
url: jdbc:mysql://localhost:3306/test
username: root
password: 123456
```
5. 在代码中使用@Value注解获取配置属性,如:
```
@Value("${server.port}")
private int port;
```
这样就可以根据不同的环境加载不同的配置文件,实现多环境配置。
### 回答2:
Spring Boot中的多环境配置主要通过yml文件来实现。在项目的src/main/resources目录下,可以创建多个不同环境的application.yml文件,如application-dev.yml、application-prod.yml等。这些文件分别对应不同的开发环境和生产环境。
在application.yml文件中,可以指定默认的配置,即所有环境都会生效的共享配置。而在不同环境的yml文件中,可以覆盖或添加特定环境下的配置。
在启动应用程序时,可以通过指定不同的spring.profiles.active属性值来选择使用哪个环境的配置。比如,可以通过在命令行中使用--spring.profiles.active=dev来指定使用开发环境的配置。
使用多环境配置可以让我们方便地切换不同的开发、测试和生产环境。在开发阶段,可以使用开发环境的配置,方便调试和开发。而在部署到生产环境时,可以使用生产环境的配置,确保应用程序的稳定性和安全性。
除了通过yml文件来配置,还可以通过编程的方式来获取多环境配置。Spring Boot提供了Environment接口和@Value注解来实现这一点。通过使用这些功能,我们可以根据当前的环境来动态获取配置值。
总而言之,Spring Boot通过yml文件和编程方式来实现多环境配置,让我们能够方便地管理不同环境下的配置,提高了开发和部署的灵活性和效率。
### 回答3:
Spring Boot 是一种框架,可以帮助开发者快速构建独立、可运行的、生产级别的 Spring 应用程序。它提供了许多便利的功能,包括多环境配置。
在 Spring Boot 中,我们可以使用 YAML(YAML Ain't Markup Language)格式的配置文件来管理不同的环境配置。通过在 `application.yml` 配置文件中定义不同的配置项,我们可以在不同环境下使用不同的配置值。
首先,我们需要创建一个 `application.yml` 文件,并在其中定义共享的配置项,例如数据库连接、服务器端口等。然后,我们可以为每个不同的环境创建独立的配置文件,例如 `application-dev.yml`、`application-test.yml`、`application-prod.yml` 等。
接下来,我们可以在 `application.yml` 文件中使用 `spring.profiles.include` 属性来指定要包含的环境配置文件,例如:
```yaml
spring:
profiles:
include: dev
```
这样,当我们启动应用程序时,Spring Boot 将自动加载 `application.yml` 和 `application-dev.yml` 两个文件中的配置项。
如果我们想切换到其他环境,可以通过在启动命令中添加 `spring.profiles.active` 参数来指定要使用的环境配置文件,例如:
```shell
java -jar myapp.jar --spring.profiles.active=test
```
这样,Spring Boot 将加载 `application.yml` 和 `application-test.yml` 两个文件中的配置项,并覆盖相同名称的配置项。
总之,通过使用 YAML 格式的配置文件和 Spring Boot 的多环境配置功能,我们可以轻松管理不同环境下的配置,并且通过命令行参数来切换不同的环境。这为我们开发和部署应用程序提供了极大的便利性和灵活性。
Springboot 切换配置文件
在Spring Boot中,您可以通过切换配置文件来为您的应用程序提供不同的配置。默认情况下,Spring Boot会加载名为`application.properties`或`application.yml`的配置文件。如果您想使用其他的配置文件,可以按照以下步骤进行操作:
1. 创建您需要的配置文件,例如`application-dev.properties`或`application-prod.yml`,并将其放在您的项目中的`src/main/resources`目录下。
2. 在`application.properties`或`application.yml`文件中设置`spring.profiles.active`属性,将其设置为您要使用的配置文件的名称,例如:
```
spring.profiles.active=dev
```
这将告诉Spring Boot使用名为`application-dev.properties`或`application-dev.yml`文件中的配置。
3. 重新启动您的应用程序,它将使用指定的配置文件。
注意:如果您同时指定了多个配置文件,则后面的配置文件中的属性将覆盖前面的配置文件中的属性。
相关推荐
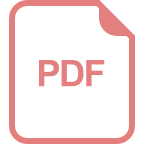
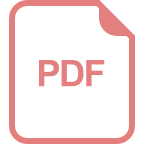
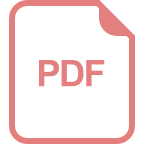
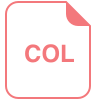











