java源代码打开摄像头_使用Java调用摄像头进行拍照源码
时间: 2023-12-30 13:05:31 浏览: 171
以下是使用Java调用摄像头进行拍照的示例代码:
```java
import java.awt.Dimension;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import com.github.sarxos.webcam.Webcam;
public class WebcamCaptureExample extends JPanel implements Runnable {
private static final long serialVersionUID = 1L;
private Webcam webcam = null;
private BufferedImage image = null;
public WebcamCaptureExample() {
super();
setPreferredSize(new Dimension(640, 480));
webcam = Webcam.getDefault();
webcam.setViewSize(new Dimension(640, 480));
}
@Override
public void run() {
while (true) {
try {
image = webcam.getImage();
repaint();
Thread.sleep(50);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
@Override
protected void paintComponent(java.awt.Graphics g) {
super.paintComponent(g);
if (image != null) {
g.drawImage(image, 0, 0, getWidth(), getHeight(), this);
}
}
public void start() {
Thread t = new Thread(this);
t.setDaemon(true);
t.start();
}
public void stop() {
webcam.close();
}
public static void main(String[] args) throws IOException {
WebcamCaptureExample example = new WebcamCaptureExample();
JFrame window = new JFrame("Webcam Capture Example");
window.add(example);
window.pack();
window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
window.setVisible(true);
example.start();
// 保存图片到文件
BufferedImage image = example.image;
File file = new File("webcam_capture.png");
ImageIO.write(image, "png", file);
example.stop();
}
}
```
该示例程序使用了 [Webcam Capture API](https://github.com/sarxos/webcam-capture) 来获取摄像头图像,需要引入对应的库文件。
程序的主要流程如下:
1. 获取默认摄像头对象并设置摄像头视野大小为640x480。
2. 实现Runnable接口,启动一个线程来获取摄像头图像并通过repaint()方法刷新面板。
3. 实现paintComponent()方法,在面板上绘制摄像头图像。
4. 启动线程,并在主线程中保存最后一帧摄像头图像到文件。
5. 在程序结束时关闭摄像头。
注意:在使用该示例代码之前,请先确保电脑上已经安装了摄像头并且能够正常使用。
阅读全文
相关推荐
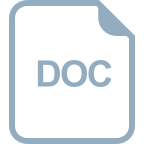
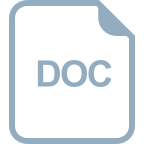
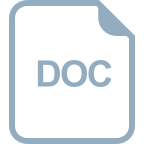
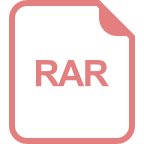
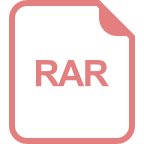
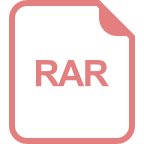
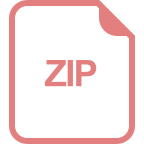
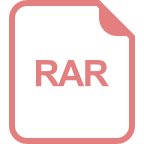
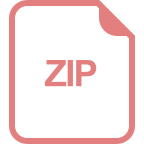
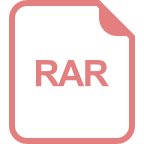
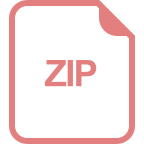
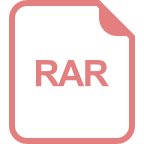
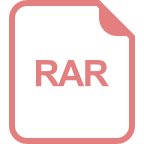
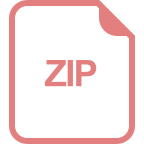
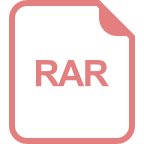
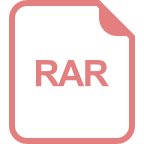
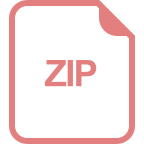
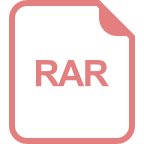
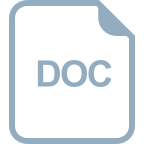