写一个requests爬取https://www.fjmotor.com.cn/allnews_list/tpid_10.html该网站的代码
时间: 2023-11-30 16:05:24 浏览: 73
好的,以下是使用Python的requests库爬取 https://www.fjmotor.com.cn/allnews_list/tpid_10.html 网站的代码:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.fjmotor.com.cn/allnews_list/tpid_10.html"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
# 示例:获取所有新闻的标题和链接
news_list = soup.find_all("div", class_="newslist-item")
for news in news_list:
title = news.find("a").text.strip()
link = news.find("a")["href"]
print(title, link)
```
上述代码首先使用requests库发送get请求,获取网页的HTML代码,然后使用BeautifulSoup库解析HTML代码。接着,我们可以通过BeautifulSoup提供的find和find_all方法查找网页中的元素,进而获取我们需要的数据。
以上代码仅供参考,实际应用时需要根据实际情况进行修改。
相关问题
需要完整的代码,比如爬取https://www.enread.com/news/business/list_188.html
为了完整地提供从URL https://www.enread.com/news/business/list_188.html 爬取数据的代码示例,我们需要使用Python的`requests`库来发送HTTP请求,`BeautifulSoup`库来解析HTML内容,以及`pandas`库来处理数据。由于实际网站结构可能会变化,下面是一个基本的模板,假设文章标题位于`<h2>`标签内,链接位于`<a>`标签的href属性中:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 定义函数来获取单页新闻列表
def get_business_news(url):
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 发送GET请求
response = requests.get(url, headers=headers)
# 检查请求是否成功
if response.status_code == 200:
# 解析HTML内容
soup = BeautifulSoup(response.text, 'lxml')
# 提取新闻标题和链接
articles = []
for article in soup.find_all('div', class_='news-item'):
title = article.h2.text.strip() if article.h2 else ''
link = article.find('a')['href'] if article.a else None
articles.append({'Title': title, 'Link': link})
# 转换为DataFrame
news_df = pd.DataFrame(articles)
return news_df
else:
print(f"Failed to fetch the page. Status code: {response.status_code}")
# 爬取指定页面
url = "https://www.enread.com/news/business/list_188.html"
news_page = get_business_news(url)
# 打印前几条数据
print(news_page.head())
# 存储数据到CSV文件
news_page.to_csv('business_news.csv', index=False)
```
注意,这个代码只是一个基本的起点,实际应用时可能需要考虑反爬虫策略(如验证码、登录验证),以及网页布局的变化。此外,如果你遇到权限问题或其他错误,可能需要联系网站管理员寻求解决方案,或者查看网站的开发者文档了解正确的抓取规则。
使用requests库爬取https://www.xynu.edu.cn/
好的,以下是使用requests库爬取https://www.xynu.edu.cn/的示例代码:
```python
import requests
url = 'https://www.xynu.edu.cn/'
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print('请求失败')
```
这段代码会发送一个GET请求到https://www.xynu.edu.cn/,并将响应内容打印出来。如果请求成功,响应状态码会是200,否则就会输出"请求失败"。你可以根据自己的需要对这段代码进行修改和扩展。
阅读全文
相关推荐
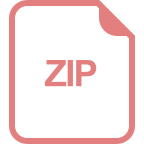
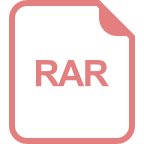
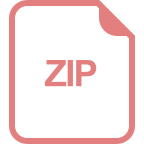













