linux C语言实现将域名转换为IP地址
时间: 2024-04-23 12:21:43 浏览: 107
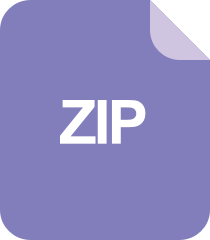
纯C语言实现DNS服务端与客户端程序
在 Linux C 语言中,可以使用 getaddrinfo 函数将域名转换为 IP 地址。
具体实现步骤如下:
1. 引入头文件:
```
#include <sys/types.h>
#include <sys/socket.h>
#include <netdb.h>
#include <arpa/inet.h>
```
2. 定义 getaddrinfo() 函数所需的结构体:
```
struct addrinfo hints, *res;
int status;
char ipstr[INET6_ADDRSTRLEN];
```
3. 初始化 hints 结构体:
```
memset(&hints, 0, sizeof hints);
hints.ai_family = AF_UNSPEC; // AF_INET 或 AF_INET6 均可
hints.ai_socktype = SOCK_STREAM; // TCP
```
4. 调用 getaddrinfo() 函数:
```
status = getaddrinfo("www.example.com", NULL, &hints, &res);
```
其中 "www.example.com" 是需要转换的域名。
5. 遍历返回的地址列表,并将其转换为 IP 地址:
```
struct addrinfo *p;
void *addr;
for(p = res; p != NULL; p = p->ai_next) {
if (p->ai_family == AF_INET) { // IPv4
struct sockaddr_in *ipv4 = (struct sockaddr_in *)p->ai_addr;
addr = &(ipv4->sin_addr);
} else { // IPv6
struct sockaddr_in6 *ipv6 = (struct sockaddr_in6 *)p->ai_addr;
addr = &(ipv6->sin6_addr);
}
// 将 IP 地址转换为字符串
inet_ntop(p->ai_family, addr, ipstr, sizeof ipstr);
}
```
其中,inet_ntop() 函数将 IP 地址转换为字符串形式,ipstr 变量即为转换后的 IP 地址。
6. 释放内存:
```
freeaddrinfo(res);
```
完整代码如下:
```
#include <sys/types.h>
#include <sys/socket.h>
#include <netdb.h>
#include <arpa/inet.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
struct addrinfo hints, *res;
int status;
char ipstr[INET6_ADDRSTRLEN];
memset(&hints, 0, sizeof hints);
hints.ai_family = AF_UNSPEC;
hints.ai_socktype = SOCK_STREAM;
status = getaddrinfo("www.example.com", NULL, &hints, &res);
if (status != 0) {
fprintf(stderr, "getaddrinfo error: %s\n", gai_strerror(status));
exit(1);
}
struct addrinfo *p;
void *addr;
for(p = res; p != NULL; p = p->ai_next) {
if (p->ai_family == AF_INET) { // IPv4
struct sockaddr_in *ipv4 = (struct sockaddr_in *)p->ai_addr;
addr = &(ipv4->sin_addr);
} else { // IPv6
struct sockaddr_in6 *ipv6 = (struct sockaddr_in6 *)p->ai_addr;
addr = &(ipv6->sin6_addr);
}
inet_ntop(p->ai_family, addr, ipstr, sizeof ipstr);
printf("IP address: %s\n", ipstr);
}
freeaddrinfo(res);
return 0;
}
```
阅读全文
相关推荐
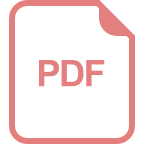
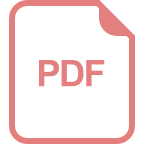















