#include <iostream> using namespace std; #include <iomanip> #include <cstring> //student.h class Student { public: Student(char *); ~Student(); void displayGrades(); void addGrade(int); static int getNumStudents(); private: int grades; char *name; int idNum; static int numStudents = 0; } //student.cpp static int numStudents = 0; Student::Student(char *nPtr) { grades= 0; name=new char[strlen(nPtr)+1]; strcpy(name, nPtr); numStudents++; cout<<"A student has been added"<<endl; } Student::~Student() { cout<<name<<" has been deleted"<<endl; delete name; numStudents--; } void Student::displayGrades() { cout<<"Here are the grades for "<<name <<endl; cout<<setw(5)<<grades; cout<<endl<<endl; } void Student::addGrade(int grade) { this->grades=grade; return ; } static int Student::getNumStudents() { return numStudents; } //debug.cpp int main() { cout<<"There are currently "<<Student::getNumStudents()<<" students" <<endl<<endl; Student s1Ptr = new Student("Student 1") ; s1Ptr->addGrade(100); s1Ptr->displayGrades(); Student *s2Ptr = new Student ("Student 2"); s2Ptr->addGrade(83); s2Ptr->displayGrades(); const Student s3("Student 3"); s3.addGrade(62); s3.displayGrades(); cout<<"There are currently "<<getNumStudents()<<" students"<<endl<<endl; delete s2Ptr; delete s1Ptr; return 0; }改正语法错误使其编译成攻
时间: 2024-03-20 08:44:53 浏览: 17
这段代码有几个语法错误:
1. 在类定义中,`static int numStudents = 0;`不能在这里初始化静态成员变量。
2. 在类定义中,需要在函数声明前加上 `Student::`,比如 `static int Student::getNumStudents();`。
3. 在 `Student` 构造函数中,应该用 `new` 分配内存给 `name`。
4. 在 `Student` 析构函数中,应该用 `delete[]` 释放 `name` 分配的内存。
5. 在 `Student::addGrade` 函数中,不需要 `return;`。
6. 在 `main` 函数中,需要用 `new` 分配内存给 `s1Ptr`,并且不能用 `const` 修饰 `s3`。
修改后的代码如下:
```
#include <iostream>
#include <iomanip>
#include <cstring>
using namespace std;
// student.h
class Student {
public:
Student(char *);
~Student();
void displayGrades();
void addGrade(int);
static int getNumStudents();
private:
int grades;
char *name;
int idNum;
static int numStudents;
};
// student.cpp
int Student::numStudents = 0;
Student::Student(char *nPtr) {
grades= 0;
name = new char[strlen(nPtr)+1];
strcpy(name, nPtr);
numStudents++;
cout << "A student has been added" << endl;
}
Student::~Student() {
cout << name << " has been deleted" << endl;
delete[] name;
numStudents--;
}
void Student::displayGrades() {
cout << "Here are the grades for " << name << endl;
cout << setw(5) << grades;
cout << endl << endl;
}
void Student::addGrade(int grade) {
this->grades = grade;
}
int Student::getNumStudents() {
return numStudents;
}
// debug.cpp
int main() {
cout << "There are currently " << Student::getNumStudents() << " students" << endl << endl;
Student *s1Ptr = new Student("Student 1");
s1Ptr->addGrade(100);
s1Ptr->displayGrades();
Student *s2Ptr = new Student("Student 2");
s2Ptr->addGrade(83);
s2Ptr->displayGrades();
Student s3("Student 3");
s3.addGrade(62);
s3.displayGrades();
cout << "There are currently " << Student::getNumStudents() << " students" << endl << endl;
delete s2Ptr;
delete s1Ptr;
return 0;
}
```
相关推荐
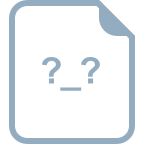















