C++通过CIP传输float类型数据代码实例
时间: 2023-07-15 07:11:13 浏览: 71
CIP是一种工业通信协议,用于在PLC和其他设备之间进行通信。在C++中,我们可以使用第三方库来实现通过CIP传输float类型数据。以下是使用OpenOPC库实现CIP通信的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <openopc.h>
int main() {
// 创建OpenOPC客户端实例
Opc::Client opc("localhost");
// 连接到CIP服务器
opc.connect("CIP", "192.168.1.100");
// 准备要传输的数据
float data = 3.14;
// 将float类型数据转换为字节数组
std::vector<unsigned char> bytes(sizeof(float));
std::memcpy(bytes.data(), &data, sizeof(float));
// 发送数据到CIP服务器
opc.write("Device1.Tag1", bytes);
// 断开CIP服务器连接
opc.disconnect();
return 0;
}
```
在上面的示例中,我们使用了OpenOPC库来连接到CIP服务器,并通过opc.write函数向指定的设备和标签发送数据。在发送数据之前,我们将float类型的数据转换为字节数组,以便在网络上传输。
相关问题
C++实现CIP传输float类型实例
CIP(Common Industrial Protocol)是一种在工业控制系统中广泛使用的通信协议。在CIP中传输float类型的数据需要将其转换为字节序列,并采用Big-Endian字节序传输。
以下是一个示例代码实现,在此我们假设要传输的float类型数据为“value”:
```c++
#include <iostream>
#include <cstring>
#include <arpa/inet.h> // for htons and htonl
using namespace std;
int main() {
float value = 3.14159f;
uint32_t netValue;
// 将float类型数据转换为Big-Endian字节序
netValue = htonl(*(uint32_t*)&value);
// 将字节序列发送到对应的接收方
// ...
// 接收方将收到的字节序列转换为float类型数据
float receivedValue;
receivedValue = *(float*)&(ntohl(netValue));
cout << "value: " << value << endl;
cout << "received value: " << receivedValue << endl;
return 0;
}
```
在上述代码中,我们使用了htonl和ntohl函数将float类型数据转换为Big-Endian字节序并传输,同时在接收方将收到的字节序列转换为float类型数据。需要注意的是,这种方式存在精度损失的问题,因为float类型数据只能精确表示一定范围内的小数。因此,在实际应用中需要根据具体场景选择合适的数据类型和传输方式。
C++实现CIP传输float数据类型
CIP(Common Industrial Protocol)是一种常用于工业自动化领域的通信协议,用于设备间的数据通信。在CIP协议中,float数据类型的传输是通过将数据转换为4个字节(32位)的二进制表示来完成的。下面是一个简单的C++程序示例,演示如何将一个float数据类型转换为4个字节表示并通过CIP协议传输:
```
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
float f = 3.14f;
char buffer[4];
memcpy(buffer, &f, sizeof(f)); // 将float类型的数据转换为4个字节的二进制表示
// 将buffer中的数据通过CIP协议传输
return 0;
}
```
在上面的示例中,我们使用了C++中的`memcpy`函数将float类型的数据转换为4个字节的二进制表示,并将其存储在一个字符数组中(`buffer`变量)。接下来,我们可以将这个字符数组通过CIP协议传输到其他设备中。需要注意的是,CIP协议的具体实现可能因设备不同而有所差异,因此具体的传输方式可能需要根据实际情况进行调整。
相关推荐
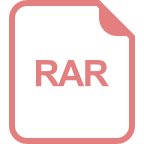
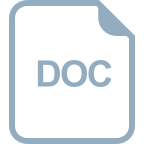












