dijkstra matlab编程
时间: 2023-08-31 19:09:29 浏览: 84
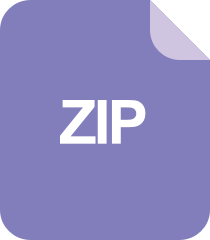
用matlab实现dijkstra算法
Dijkstra算法是一种最短路径算法,用于在带权图中找到从一个节点到另一个节点的最短路径。下面是一个使用Matlab实现Dijkstra算法的示例代码:
```matlab
function [dist, path] = dijkstra(graph, start, goal)
% DIJKSTRA Calculates the shortest path in a graph using Dijkstra's algorithm
% graph - a weighted adjacency matrix representing the graph
% start - the index of the start node
% goal - the index of the goal node
% dist - a vector containing the minimum distance from the start node to each node in the graph
% path - a vector containing the path from the start node to the goal node
n = size(graph, 1); % number of nodes in the graph
dist = Inf(1, n); % initialize distances to infinity
dist(start) = 0; % distance from start node to itself is 0
visited = false(1, n); % keep track of visited nodes
path = zeros(1, n); % initialize path
for i = 1:n-1 % loop through all nodes except the last one
% select the unvisited node with the smallest distance
[~, current] = min(dist .* ~visited);
visited(current) = true; % mark node as visited
% update distances and paths for adjacent nodes
neighbors = find(graph(current, :));
for j = 1:length(neighbors)
neighbor = neighbors(j);
if ~visited(neighbor)
new_dist = dist(current) + graph(current, neighbor);
if new_dist < dist(neighbor)
dist(neighbor) = new_dist;
path(neighbor) = current;
end
end
end
end
% construct path from start to goal node
node = goal;
while node ~= start
path(node) = path(node) + 1;
node = path(node);
end
path = fliplr(path);
end
```
该代码使用了一个邻接矩阵来表示带权图,其中图中的每个节点都被表示为一个数字索引。在执行算法时,它从起始节点开始,通过遍历相邻节点来计算到每个节点的最短距离,并记录路径。最后,它构造了从起始节点到目标节点的最短路径。
阅读全文
相关推荐
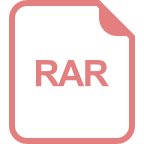
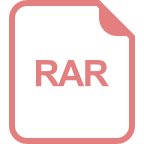
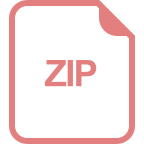
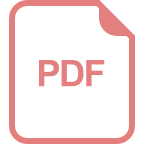
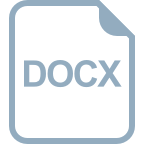
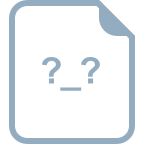
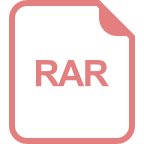
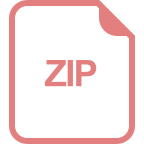



