设计异形迷宫,不同于传统的方形迷宫,异形迷宫 中每个迷宫单元是由相同的多边形组成,如图,是 一个六边形迷宫,每一个单元格与其它单元格最多 有六个通道可以连接。 1)自动生成并走出迷宫。 2)迷宫单元的边数由用户输入确定。 c++
时间: 2024-06-08 08:08:34 浏览: 182
实现一个异形迷宫需要以下步骤:
1. 定义迷宫单元:一个迷宫单元包含多个相同的多边形,每个多边形有多个顶点和边。
2. 构建迷宫:我们可以使用递归算法或者随机算法来构建异形迷宫。递归算法是基于分治思想的,将迷宫不断分解为更小的区域,直到达到基础情况。随机算法则是随机地打通迷宫中的通道,直到所有区域都连通为止。
3. 寻找迷宫路径:我们可以使用深度优先搜索或者广度优先搜索来寻找迷宫中的路径。这里我们以深度优先搜索为例。
下面是一份C++代码实现:
```cpp
#include <iostream>
#include <vector>
#include <stack>
#include <cstdlib>
#include <ctime>
using namespace std;
// 定义多边形
struct Polygon {
vector<pair<int, int>> vertices; // 多边形的顶点
vector<pair<int, int>> edges; // 多边形的边
};
// 定义迷宫单元
struct Cell {
vector<Polygon> polygons; // 迷宫单元包含的多边形
vector<Cell*> neighbors; // 迷宫单元相邻的单元格
bool visited; // 是否已访问过
};
// 定义异形迷宫
class Maze {
public:
Maze(int n) {
srand((unsigned)time(NULL));
int num_edges = n;
int num_polygons = rand() % (n * n) + n; // 随机生成多边形的数量
for (int i = 0; i < num_polygons; ++i) {
Polygon polygon;
int num_vertices = rand() % (num_edges - 2) + 3; // 随机生成多边形的顶点数
for (int j = 0; j < num_vertices; ++j) {
pair<int, int> vertex(rand() % 10, rand() % 10); // 随机生成顶点的坐标
polygon.vertices.push_back(vertex);
if (j > 0) {
polygon.edges.push_back(make_pair(j - 1, j));
}
}
polygon.edges.push_back(make_pair(num_vertices - 1, 0));
polygons_.push_back(polygon);
}
// 构建迷宫单元
for (int i = 0; i < num_polygons; ++i) {
Cell* cell = new Cell;
cell->polygons.push_back(polygons_[i]);
cells_.push_back(cell);
}
// 打通迷宫中的通道
for (int i = 0; i < num_polygons; ++i) {
int num_neighbors = rand() % 6;
for (int j = 0; j < num_neighbors; ++j) {
int index = rand() % num_polygons;
if (index != i) {
cells_[i]->neighbors.push_back(cells_[index]);
}
}
}
}
void solve() {
stack<Cell*> s;
s.push(cells_[0]);
while (!s.empty()) {
Cell* cell = s.top();
s.pop();
if (!cell->visited) {
cell->visited = true;
if (cell == cells_.back()) {
cout << "Solved!" << endl;
return;
}
for (Cell* neighbor : cell->neighbors) {
s.push(neighbor);
}
}
}
cout << "No solution!" << endl;
}
private:
vector<Polygon> polygons_;
vector<Cell*> cells_;
};
int main() {
Maze maze(6);
maze.solve();
return 0;
}
```
这里使用随机算法生成异形迷宫。首先随机生成多边形的数量和顶点数,然后构建迷宫单元。接着打通迷宫中的通道,在每个单元格中随机选择相邻的单元格。最后使用深度优先搜索寻找迷宫路径。
阅读全文
相关推荐
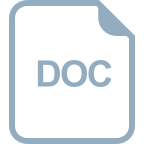
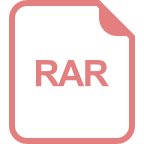








