python 根据坐标绘制折线图
时间: 2023-09-13 14:10:00 浏览: 99
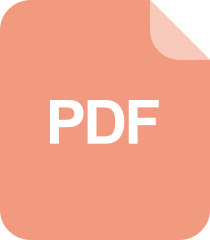
python使用matplotlib绘制折线图教程
使用Python可以使用matplotlib库来绘制折线图。下面是一个示例代码,可以根据给定的x和y坐标绘制折线图:
```python
import matplotlib.pyplot as plt
# 绘制的数据
x = [1, 2, 3, 4, 5]
y = [3, 5, 6, 8, 4]
# 绘制折线图
plt.plot(x, y)
# 添加标题和坐标轴标签
plt.title("Line Chart")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
# 显示图形
plt.show()
```
在上面的代码中,`x`和`y`分别表示要绘制的数据点的x坐标和y坐标。`plt.plot(x, y)`用于绘制折线图。可以使用`plt.title()`、`plt.xlabel()`和`plt.ylabel()`函数来添加标题和坐标轴标签。最后使用`plt.show()`将图形显示出来。
如果需要绘制多条折线,只需要在`plt.plot()`中传入多组`x`和`y`坐标即可。
阅读全文
相关推荐
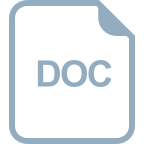
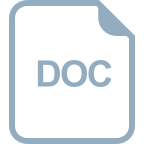



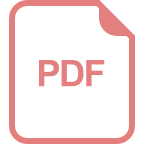
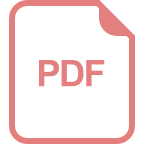
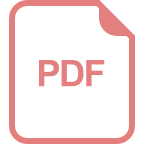









