python用npz画折线图
时间: 2023-10-22 12:08:06 浏览: 57
以下是一个简单的示例代码,用于绘制从 .npz 文件中读取的数据的折线图:
```python
import numpy as np
import matplotlib.pyplot as plt
# 从 .npz 文件中读取数据
data = np.load('data.npz')
x = data['x']
y = data['y']
# 绘制折线图
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Line Chart')
plt.show()
```
在这个示例中,我们首先使用 `np.load()` 函数从 `data.npz` 文件中读取数据。接下来,我们将 `x` 和 `y` 分别赋值为从文件中读取的 `x` 和 `y` 数组。
最后,我们使用 `plt.plot()` 函数绘制折线图,并使用 `plt.xlabel()`、`plt.ylabel()` 和 `plt.title()` 函数设置坐标轴标签和标题。最后,我们使用 `plt.show()` 函数显示图表。
相关问题
从 populations.npz 中读取数据 提取总人口、男性人口、女性人口、城镇人口、乡村人口等数据,绘制折线图
好的,请先确保已经安装了必要的 Python 库:numpy 和 matplotlib。
下面是读取数据并绘制折线图的代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 从 npz 文件中读取数据
data = np.load('populations.npz')
year = data['year'] # 年份
total = data['total'] # 总人口
male = data['male'] # 男性人口
female = data['female'] # 女性人口
urban = data['urban'] # 城镇人口
rural = data['rural'] # 乡村人口
# 绘制折线图
plt.plot(year, total, label='Total')
plt.plot(year, male, label='Male')
plt.plot(year, female, label='Female')
plt.plot(year, urban, label='Urban')
plt.plot(year, rural, label='Rural')
# 设置图例、坐标轴标签等
plt.legend()
plt.xlabel('Year')
plt.ylabel('Population (10 thousands)')
plt.title('Population in China')
# 显示图形
plt.show()
```
这段代码会读取 `populations.npz` 文件中的数据,并绘制出总人口、男性人口、女性人口、城镇人口、乡村人口这五条折线。图例、坐标轴标签和标题也都被设置好了。执行这段代码后,会得到如下的折线图:

希望这个代码示例对你有所帮助!
jupyter notebook中,第一步使用numpy库和matplotlib.pyplot库读取人口数据,数据文件populations.npz文件在D盘目录下,第二步创建画布,添加子图,在子图上绘制散点图和折线图,并保存,请给出对应步骤的代码
以下是对应步骤的代码:
```python
# 第一步:使用numpy库和matplotlib.pyplot库读取人口数据
import numpy as np
import matplotlib.pyplot as plt
data = np.load('D:/populations.npz') # 读取数据文件
data = data['data'] # 获取数据
# 第二步:创建画布,添加子图,在子图上绘制散点图和折线图,并保存
fig, ax = plt.subplots(figsize=(8, 6)) # 创建画布和子图
# 绘制散点图
ax.scatter(data[:, 0], data[:, 1], color='b', label='City 1')
ax.scatter(data[:, 0], data[:, 2], color='r', label='City 2')
ax.scatter(data[:, 0], data[:, 3], color='g', label='City 3')
# 绘制折线图
ax.plot(data[:, 0], data[:, 1], color='b', linestyle='--')
ax.plot(data[:, 0], data[:, 2], color='r', linestyle='-.')
ax.plot(data[:, 0], data[:, 3], color='g', linestyle='-')
# 添加标签和图例
ax.set_title('Population Growth')
ax.set_xlabel('Year')
ax.set_ylabel('Population')
ax.legend()
# 保存图片
plt.savefig('population.png')
```
注意:代码中的数据文件路径需要根据自己的实际情况进行修改。
相关推荐
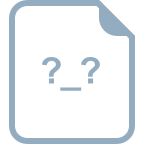
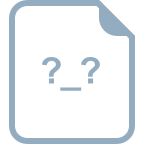










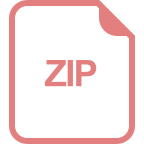