SSM框架展示HDFS文件列表,上传文件,删除文件,下载文件前后端代码
时间: 2023-09-26 09:06:47 浏览: 57
### 回答1:
这里提供一个基于SpringMVC和Hadoop API开发的展示HDFS文件列表、上传文件、删除文件、下载文件的简单示例代码。
1. 展示HDFS文件列表的后端代码
```java
package com.example.demo.controller;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileStatus;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
@Controller
public class HDFSController {
private final String HDFS_URL = "hdfs://localhost:9000";
@GetMapping("/list")
public String listHDFS(Model model) throws URISyntaxException, IOException {
Configuration configuration = new Configuration();
FileSystem fileSystem = FileSystem.get(new URI(HDFS_URL), configuration);
Path path = new Path("/");
FileStatus[] status = fileSystem.listStatus(path);
model.addAttribute("files", status);
return "list";
}
}
```
2. 展示HDFS文件列表的前端代码
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>HDFS文件列表</title>
</head>
<body>
<h1>HDFS文件列表</h1>
<table>
<thead>
<tr>
<th>文件名</th>
<th>文件大小</th>
<th>修改时间</th>
</tr>
</thead>
<tbody>
<tr th:each="file : ${files}">
<td th:text="${file.getPath().getName()}"></td>
<td th:text="${#numbers.formatDecimal(file.getLen()/1024/1024,1,'POINT',2)} + ' MB'"></td>
<td th:text="${#dates.format(file.getModificationTime(),'yyyy-MM-dd HH:mm:ss')}"></td>
</tr>
</tbody>
</table>
</body>
</html>
```
3. 上传文件的后端代码
```java
package com.example.demo.controller;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
@Controller
public class HDFSController {
private final String HDFS_URL = "hdfs://localhost:9000";
@PostMapping("/upload")
public String uploadHDFS(@RequestParam("file") MultipartFile file) throws URISyntaxException, IOException {
Configuration configuration = new Configuration();
FileSystem fileSystem = FileSystem.get(new URI(HDFS_URL), configuration);
String fileName = file.getOriginalFilename();
Path path = new Path("/" + fileName);
fileSystem.copyFromLocalFile(new Path(file.getOriginalFilename()), path);
return "redirect:/list";
}
}
```
4. 上传文件的前端代码
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>上传文件</title>
</head>
<body>
<h1>上传文件</h1>
<form method="post" action="/upload" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="上传">
</form>
</body>
</html>
```
5. 删除文件的后端代码
```java
package com.example.demo.controller;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
@Controller
public class HDFSController {
private final String HDFS_URL = "hdfs://localhost:9000";
@GetMapping("/delete/{fileName}")
public String deleteHDFS(@PathVariable String fileName) throws URISyntaxException, IOException {
Configuration configuration = new Configuration();
FileSystem fileSystem = FileSystem.get(new URI(HDFS_URL), configuration);
Path path = new Path("/" + fileName);
fileSystem.delete(path, false);
return "redirect:/list";
}
}
```
6. 下载文件的后端代码
```java
package com.example.demo.controller;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.springframework.stereotype.Controller;
import org.springframework.util.StreamUtils;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.net.URISyntaxException;
@Controller
public class HDFSController {
private final String HDFS_URL = "hdfs://localhost:9000";
@GetMapping("/download/{fileName}")
public void downloadHDFS(@PathVariable String fileName, HttpServletResponse response) throws URISyntaxException, IOException {
Configuration configuration = new Configuration();
FileSystem fileSystem = FileSystem.get(new URI(HDFS_URL), configuration);
Path path = new Path("/" + fileName);
InputStream inputStream = fileSystem.open(path);
response.setHeader("Content-Disposition", "attachment; filename=" + fileName);
StreamUtils.copy(inputStream, response.getOutputStream());
}
}
```
注意:需要在项目中引入hadoop-core和spring-web依赖。以上代码仅为示例,需要根据实际情况进行修改。
### 回答2:
SSM框架(Spring+SpringMVC+MyBatis)是一种常用的Java Web开发框架,可以用于构建大型、可扩展的企业级应用程序。下面是使用SSM框架展示HDFS文件列表、上传文件、删除文件、下载文件的前后端代码示例。
1. 前端代码:
展示HDFS文件列表:
```html
<html>
<head>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(function(){
$.ajax({
url: "getFiles",
type: "GET",
success: function(data){
var files = JSON.parse(data);
var ul = $("<ul>");
for(var i=0; i<files.length; i++){
var li = $("<li>").text(files[i]);
ul.append(li);
}
$("#fileList").append(ul);
}
});
});
</script>
</head>
<body>
<div id="fileList"></div>
</body>
</html>
```
上传文件:
```html
<html>
<head>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(function(){
$("#uploadForm").submit(function(e) {
e.preventDefault();
var formData = new FormData(this);
$.ajax({
url: "uploadFile",
type: "POST",
data: formData,
processData: false,
contentType: false,
success: function(){
alert("文件上传成功!");
}
});
});
});
</script>
</head>
<body>
<form id="uploadForm" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="上传">
</form>
</body>
</html>
```
删除文件:
```html
<html>
<head>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(function(){
$(".deleteBtn").click(function(){
var fileName = $(this).attr("data-filename");
$.ajax({
url: "deleteFile",
type: "POST",
data: {
fileName: fileName
},
success: function(){
alert("文件删除成功!");
}
});
});
});
</script>
</head>
<body>
<ul>
<li><span>file1.txt</span> <button class="deleteBtn" data-filename="file1.txt">删除</button></li>
<li><span>file2.txt</span> <button class="deleteBtn" data-filename="file2.txt">删除</button></li>
<li><span>file3.txt</span> <button class="deleteBtn" data-filename="file3.txt">删除</button></li>
</ul>
</body>
</html>
```
下载文件:
```html
<html>
<head>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
</head>
<body>
<a href="downloadFile?fileName=file1.txt">下载文件1</a>
<a href="downloadFile?fileName=file2.txt">下载文件2</a>
<a href="downloadFile?fileName=file3.txt">下载文件3</a>
</body>
</html>
```
2. 后端代码:
展示HDFS文件列表(使用SpringMVC):
```java
@Controller
public class FileController {
@RequestMapping("/getFiles")
@ResponseBody
public String getFiles() {
// 查询HDFS文件列表的代码
List<String> files = new ArrayList<>();
files.add("file1.txt");
files.add("file2.txt");
files.add("file3.txt");
return JSON.toJSONString(files);
}
}
```
文件上传(使用SpringMVC):
```java
@Controller
public class FileController {
@RequestMapping("/uploadFile")
@ResponseBody
public void uploadFile(HttpServletRequest request, @RequestParam("file") MultipartFile file) {
String fileName = file.getOriginalFilename();
// 将文件上传到HDFS的代码
}
}
```
文件删除(使用SpringMVC):
```java
@Controller
public class FileController {
@RequestMapping("/deleteFile")
@ResponseBody
public void deleteFile(@RequestParam("fileName") String fileName) {
// 在HDFS中删除文件的代码
}
}
```
文件下载(使用SpringMVC):
```java
@Controller
public class FileController {
@RequestMapping("/downloadFile")
public void downloadFile(@RequestParam("fileName") String fileName, HttpServletResponse response) {
// 从HDFS中下载文件的代码
// 将文件内容写入response输出流
}
}
```
以上是使用SSM框架展示HDFS文件列表、上传文件、删除文件、下载文件的前后端代码。请根据实际情况修改代码中的URL、参数名等部分。在实际使用中,需要引入相关依赖、配置SSM框架的配置文件等。
### 回答3:
SSM框架是指Spring+SpringMVC+MyBatis的组合,它是一种优秀的Java开发框架,用于开发Web应用程序。下面是一个展示HDFS文件列表、上传文件、删除文件和下载文件的SSM框架的前后端代码示例。
后端代码示例:
1. 文件列表展示:
- 创建一个Controller类,处理前端请求并返回文件列表数据。
```java
@Controller
@RequestMapping("/file")
public class FileController {
@Autowired
private HadoopFileSystem hadoopFileSystem;
@RequestMapping("/list")
@ResponseBody
public List<String> getFileList() {
return hadoopFileSystem.getFileList();
}
}
```
- 创建一个HadoopFileSystem类,用于连接到HDFS并获取文件列表。
```java
@Component
public class HadoopFileSystem {
private static final String HDFS_URI = "hdfs://localhost:9000";
private static final Configuration configuration = new Configuration();
public List<String> getFileList() {
try {
FileSystem fs = FileSystem.get(URI.create(HDFS_URI), configuration);
FileStatus[] fileStatuses = fs.listStatus(new Path("/"));
List<String> fileList = new ArrayList<>();
for (FileStatus fileStatus : fileStatuses) {
if (fileStatus.isFile()) {
fileList.add(fileStatus.getPath().getName());
}
}
return fileList;
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
}
```
2. 文件上传:
- 创建一个Controller类,用于处理前端上传文件的请求。
```java
@Controller
@RequestMapping("/file")
public class FileController {
@Autowired
private HadoopFileSystem hadoopFileSystem;
@RequestMapping("/upload")
@ResponseBody
public void uploadFile(@RequestParam("file") MultipartFile file) {
hadoopFileSystem.uploadFile(file);
}
}
```
- 修改HadoopFileSystem类,添加一个上传文件的方法。
```java
@Component
public class HadoopFileSystem {
// ...
public void uploadFile(MultipartFile file) {
try {
FileSystem fs = FileSystem.get(URI.create(HDFS_URI), configuration);
InputStream inputStream = file.getInputStream();
OutputStream outputStream = fs.create(new Path("/" + file.getOriginalFilename()));
IOUtils.copyBytes(inputStream, outputStream, configuration);
fs.close();
outputStream.close();
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
3. 文件删除:
- 创建一个Controller类,用于处理前端删除文件的请求。
```java
@Controller
@RequestMapping("/file")
public class FileController {
@Autowired
private HadoopFileSystem hadoopFileSystem;
@RequestMapping("/delete")
@ResponseBody
public void deleteFile(@RequestParam("fileName") String fileName) {
hadoopFileSystem.deleteFile(fileName);
}
}
```
- 修改HadoopFileSystem类,添加一个删除文件的方法。
```java
@Component
public class HadoopFileSystem {
// ...
public void deleteFile(String fileName) {
try {
FileSystem fs = FileSystem.get(URI.create(HDFS_URI), configuration);
fs.delete(new Path("/" + fileName), true);
fs.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
4. 文件下载:
- 创建一个Controller类,用于处理前端下载文件的请求。
```java
@Controller
@RequestMapping("/file")
public class FileController {
@Autowired
private HadoopFileSystem hadoopFileSystem;
@RequestMapping("/download")
public ResponseEntity<InputStreamResource> downloadFile(@RequestParam("fileName") String fileName) {
InputStreamResource resource = hadoopFileSystem.downloadFile(fileName);
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment;filename=" + fileName)
.contentLength(resource.contentLength())
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
}
}
```
- 修改HadoopFileSystem类,添加一个下载文件的方法。
```java
@Component
public class HadoopFileSystem {
// ...
public InputStreamResource downloadFile(String fileName) {
try {
FileSystem fs = FileSystem.get(URI.create(HDFS_URI), configuration);
FSDataInputStream inputStream = fs.open(new Path("/" + fileName));
return new InputStreamResource(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
}
```
以上是一个基于SSM框架的展示HDFS文件列表、上传文件、删除文件和下载文件的前后端代码示例。注意,为了简化代码,省略了一些错误处理和其他相关的方法。在实际开发中,还需要根据实际需求进行适当的扩展和优化。
相关推荐
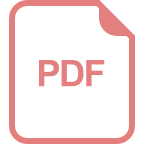
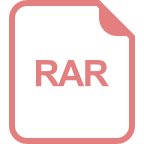



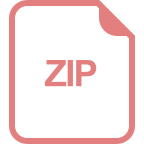
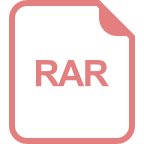
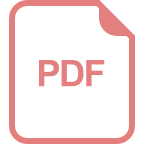
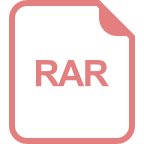
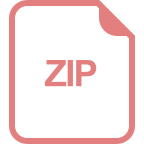
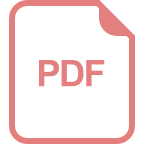
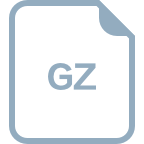
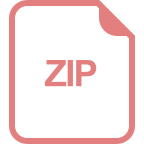