写一段Android 实现一个页面有几十个item, 每项都可以下拉选择数据
时间: 2024-03-08 21:46:37 浏览: 70
好的,下面是一个简单的 Android 代码示例,演示如何实现一个页面有几十个 item,每项都可以下拉选择数据:
在布局文件中添加一个 RecyclerView:
```xml
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
创建一个数据类,用于保存每个 item 的数据:
```kotlin
data class ItemData(val name: String, var selectedOption: String, val options: List<String>)
```
创建一个 Adapter 类,继承自 RecyclerView.Adapter,用于绑定数据和视图:
```kotlin
class ItemsAdapter(private val items: List<ItemData>) :
RecyclerView.Adapter<ItemsAdapter.ViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context)
.inflate(R.layout.item_layout, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
val itemData = items[position]
holder.nameTextView.text = itemData.name
val optionsAdapter = ArrayAdapter(
holder.itemView.context,
android.R.layout.simple_spinner_item,
itemData.options
)
optionsAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item)
holder.optionsSpinner.adapter = optionsAdapter
holder.optionsSpinner.setSelection(itemData.options.indexOf(itemData.selectedOption))
holder.optionsSpinner.onItemSelectedListener = object : AdapterView.OnItemSelectedListener {
override fun onItemSelected(
parent: AdapterView<*>?,
view: View?,
position: Int,
id: Long
) {
itemData.selectedOption = itemData.options[position]
}
override fun onNothingSelected(parent: AdapterView<*>?) {}
}
}
override fun getItemCount() = items.size
inner class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val nameTextView: TextView = itemView.findViewById(R.id.nameTextView)
val optionsSpinner: Spinner = itemView.findViewById(R.id.optionsSpinner)
}
}
```
在 Activity 或 Fragment 中,设置 RecyclerView 的 Adapter 和 LayoutManager:
```kotlin
val recyclerView = findViewById<RecyclerView>(R.id.recyclerView)
recyclerView.layoutManager = LinearLayoutManager(this)
recyclerView.adapter = ItemsAdapter(items)
```
其中,`items` 是一个包含每个 item 数据的列表。
最后,创建一个 item 的布局文件,包含一个 TextView 和一个 Spinner:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/nameTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Spinner
android:id="@+id/optionsSpinner"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
```
这样,你就可以在一个页面中创建多个 item,每个 item 都可以下拉选择数据了。用户选择的数据将保存在每个 item 对应的数据类中(在这个示例中是 `ItemData` 类)。
阅读全文
相关推荐


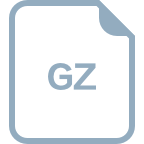












