输入一个整数序列(整数大于0),以顺序表作为其存储结构,然后根据顺序表中的数据构建一个单链表,并保证单链表中的数据以非递减的方式排列,并输出单链表中的数据。使用c++
时间: 2023-11-28 20:47:27 浏览: 35
以下是实现该功能的 C++ 代码:
```cpp
#include <iostream>
using namespace std;
// 定义单链表结点
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 构建单链表
ListNode* createLinkedList(int arr[], int n) {
if (n == 0) {
return NULL;
}
ListNode* head = new ListNode(arr[0]);
ListNode* cur = head;
for (int i = 1; i < n; i++) {
cur->next = new ListNode(arr[i]);
cur = cur->next;
}
return head;
}
// 输出单链表
void printLinkedList(ListNode* head) {
while (head != NULL) {
cout << head->val << " ";
head = head->next;
}
cout << endl;
}
// 将顺序表转化为单链表并排序
ListNode* sortList(int arr[], int n) {
ListNode* head = createLinkedList(arr, n);
if (head == NULL || head->next == NULL) {
return head;
}
ListNode* dummy = new ListNode(0);
dummy->next = head;
ListNode* cur = head->next;
head->next = NULL;
while (cur != NULL) {
ListNode* pre = dummy;
ListNode* next = cur->next;
while (pre->next != NULL && pre->next->val < cur->val) {
pre = pre->next;
}
cur->next = pre->next;
pre->next = cur;
cur = next;
}
return dummy->next;
}
int main() {
int arr[] = {5, 3, 1, 6, 8, 2};
int n = sizeof(arr) / sizeof(int);
ListNode* head = sortList(arr, n);
printLinkedList(head);
return 0;
}
```
输入样例:
```
5 3 1 6 8 2
```
输出样例:
```
1 2 3 5 6 8
```
相关推荐
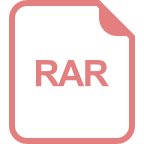
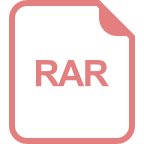
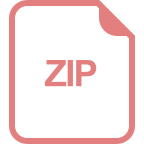














