python-opencv 阈值处理
时间: 2023-09-24 07:13:59 浏览: 94
阈值处理是一种图像处理技术,它将图像中的像素值与给定的阈值进行比较,并将它们分成两个类别:高于阈值和低于阈值。在 Python 中,使用 OpenCV 库可以实现阈值处理。
以下是使用 Python-OpenCV 进行阈值处理的步骤:
1. 导入 OpenCV 库和 NumPy 库
```python
import cv2
import numpy as np
```
2. 读取图像
```python
img = cv2.imread('image.jpg')
```
3. 将图像转换为灰度图像
```python
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
4. 对灰度图像进行阈值处理
```python
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
```
其中,`gray` 是灰度图像,`127` 是阈值,`255` 是像素值的最大值,`cv2.THRESH_BINARY` 表示二进制阈值处理。
5. 显示阈值处理后的图像
```python
cv2.imshow('thresholded image', thresh)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
完整代码如下:
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
cv2.imshow('thresholded image', thresh)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码将阈值设置为 127,像素值的最大值为 255,将灰度图像进行二进制阈值处理,并显示处理后的图像。可以根据需要调整阈值的值,以获得更好的效果。
阅读全文
相关推荐
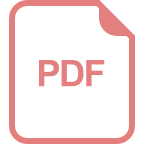
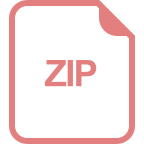
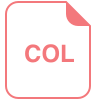
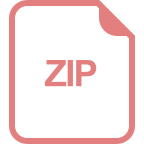
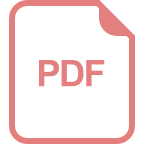
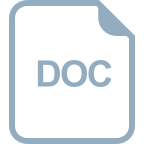
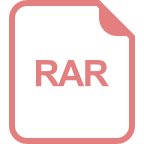
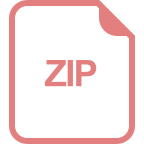
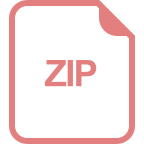
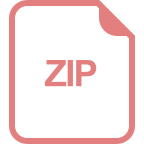
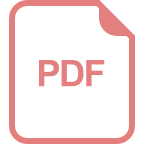
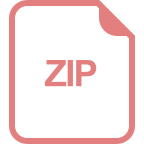
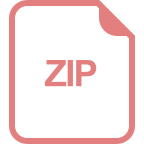



