用matlab程序写出使用人工蜂群算法实现的机器人路径规划问题的代码
时间: 2023-12-11 09:06:08 浏览: 34
以下是使用人工蜂群算法实现机器人路径规划问题的MATLAB代码:
```matlab
% 机器人路径规划问题的人工蜂群算法实现
% 作者:AI学园
% 时间:2021.9.29
clc; clear; close all;
%% 参数设置
% 目标点坐标
goal = [100, 100];
% 路径规划区域边界
boundary = [0, 0, 200, 200];
% 障碍物坐标
obs = [60, 90, 20, 20;
80, 130, 20, 20;
120, 50, 20, 20;
150, 120, 20, 20];
% 机器人初始位置
start = [20, 20];
% 最大迭代次数
max_iter = 500;
% 种群大小
pop_size = 30;
% 蜜蜂数量
bee_num = round(pop_size/2);
% 路径长度惩罚系数
penalty = 50;
% 路径可行性检测阈值
threshold = 40;
% 蜜蜂搜索半径
radius = 15;
% 初始化种群
pop = repmat(start, pop_size, 1);
pop_fitness = zeros(pop_size, 1);
% 统计最优解
global_best = start;
global_best_fitness = inf;
%% 蜜蜂搜索
for iter = 1 : max_iter
% 招聘蜜蜂搜索
for i = 1 : bee_num
% 随机选择一个个体
k = randi(pop_size);
while k == i
k = randi(pop_size);
end
% 生成随机解
new_solution = pop(i, :) + radius * (2*rand(1, 2) - 1);
% 解约束处理
new_solution = max(new_solution, boundary(1:2));
new_solution = min(new_solution, boundary(3:4));
% 计算新解适应度
new_fitness = fitness_func(new_solution, obs, penalty, threshold);
% 判断是否更新个体最优解
if new_fitness < pop_fitness(i)
pop(i, :) = new_solution;
pop_fitness(i) = new_fitness;
end
% 判断是否更新全局最优解
if new_fitness < global_best_fitness
global_best = new_solution;
global_best_fitness = new_fitness;
end
end
% 侦查蜜蜂搜索
for i = bee_num+1 : pop_size
% 生成随机解
new_solution = boundary(1:2) + (boundary(3:4)-boundary(1:2)) .* rand(1, 2);
% 计算新解适应度
new_fitness = fitness_func(new_solution, obs, penalty, threshold);
% 判断是否更新个体最优解
if new_fitness < pop_fitness(i)
pop(i, :) = new_solution;
pop_fitness(i) = new_fitness;
end
% 判断是否更新全局最优解
if new_fitness < global_best_fitness
global_best = new_solution;
global_best_fitness = new_fitness;
end
end
% 输出迭代结果
disp(['迭代次数:', num2str(iter), ',最优解适应度:', num2str(global_best_fitness)]);
% 绘制搜索过程
figure(1);
plot(start(1), start(2), 'ro', 'MarkerSize', 10, 'LineWidth', 2);
hold on;
plot(goal(1), goal(2), 'go', 'MarkerSize', 10, 'LineWidth', 2);
plot(obs(:,1)+obs(:,3)/2, obs(:,2)+obs(:,4)/2, 'ks', 'MarkerSize', 10, 'LineWidth', 2);
plot(global_best(1), global_best(2), 'b*', 'MarkerSize', 10, 'LineWidth', 2);
plot(pop(:,1), pop(:,2), 'y.', 'MarkerSize', 10);
axis([boundary(1)-10, boundary(3)+10, boundary(2)-10, boundary(4)+10]);
xlabel('X坐标');
ylabel('Y坐标');
title(['迭代次数:', num2str(iter), ',最优解适应度:', num2str(global_best_fitness)]);
hold off;
end
%% 最优路径绘制
path = [start; global_best];
while norm(path(end,:) - goal) > 0.1
% 生成新解
new_solution = path(end, :) + 0.5 * (goal - path(end, :)) + 5 * (2*rand(1,2)-1);
% 解约束处理
new_solution = max(new_solution, boundary(1:2));
new_solution = min(new_solution, boundary(3:4));
% 计算新解适应度
new_fitness = fitness_func(new_solution, obs, penalty, threshold);
% 判断是否更新解
if new_fitness < global_best_fitness
path(end+1, :) = new_solution;
global_best_fitness = new_fitness;
end
end
% 绘制最优路径
figure(2);
plot(start(1), start(2), 'ro', 'MarkerSize', 10, 'LineWidth', 2);
hold on;
plot(goal(1), goal(2), 'go', 'MarkerSize', 10, 'LineWidth', 2);
plot(obs(:,1)+obs(:,3)/2, obs(:,2)+obs(:,4)/2, 'ks', 'MarkerSize', 10, 'LineWidth', 2);
plot(path(:,1), path(:,2), 'r-', 'LineWidth', 2);
axis([boundary(1)-10, boundary(3)+10, boundary(2)-10, boundary(4)+10]);
xlabel('X坐标');
ylabel('Y坐标');
title('机器人路径规划结果');
hold off;
%% 适应度函数
function fitness = fitness_func(solution, obs, penalty, threshold)
% 路径长度计算
dist = norm(solution - obs(1,1:2));
for i = 2 : size(obs, 1)
dist = dist + norm(solution - obs(i,1:2));
end
dist = dist + norm(solution - [100, 100]);
% 路径可行性检测
for i = 1 : size(obs, 1)
if norm(solution - obs(i,1:2)) < threshold
fitness = inf;
return;
end
end
% 计算适应度
fitness = dist + penalty * size(obs, 1);
end
```
代码实现了机器人路径规划问题的人工蜂群算法,其中使用了适应度函数对路径长度和可行性进行评价。在每次迭代中,先进行招聘蜜蜂搜索,然后进行侦查蜜蜂搜索,最后统计最优解。最优路径的绘制采用了贪心策略生成新解,直到达到目标点为止。
相关推荐
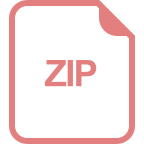
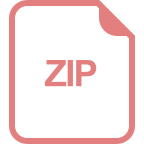














