linux设计两个程序:有名管道的读程序和写程序,要求利用有名管道实现聊天程序,每次发言后自动在后面增加当前系统时间。增加结束字符,比如最后输入“886”后结束进程。
时间: 2023-12-18 21:05:36 浏览: 18
下面是有名管道聊天程序的代码实现:
读程序(chat_read.c):
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#include <time.h>
#define FIFO_FILE "chat_fifo"
int main()
{
int fd;
char buf[1024];
time_t t;
/* 打开有名管道 */
fd = open(FIFO_FILE, O_RDONLY);
if (fd < 0) {
perror("open");
exit(1);
}
while (1) {
/* 从管道中读取数据 */
if (read(fd, buf, sizeof(buf)) > 0) {
/* 获取系统当前时间 */
t = time(NULL);
/* 将时间转换为字符串,并将时间和数据拼接在一起 */
sprintf(buf, "[%s]: %s", ctime(&t), buf);
/* 输出到屏幕上 */
printf("%s", buf);
}
/* 判断是否结束程序 */
if (strcmp(buf, "886\n") == 0)
break;
}
/* 关闭管道 */
close(fd);
return 0;
}
```
写程序(chat_write.c):
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#define FIFO_FILE "chat_fifo"
int main()
{
int fd;
char buf[1024];
/* 创建有名管道 */
if (mkfifo(FIFO_FILE, 0666) < 0) {
perror("mkfifo");
exit(1);
}
/* 打开有名管道 */
fd = open(FIFO_FILE, O_WRONLY);
if (fd < 0) {
perror("open");
exit(1);
}
while (1) {
/* 从标准输入中读取数据 */
fgets(buf, sizeof(buf), stdin);
/* 将数据写入管道中 */
write(fd, buf, strlen(buf));
/* 判断是否结束程序 */
if (strcmp(buf, "886\n") == 0)
break;
}
/* 关闭管道 */
close(fd);
/* 删除有名管道 */
unlink(FIFO_FILE);
return 0;
}
```
使用方法:
1. 在终端中进入程序所在的目录,先运行读程序(`./chat_read`);
2. 再运行写程序(`./chat_write`);
3. 在写程序的终端输入聊天内容,按回车键发送,聊天内容及时间会在读程序的终端中显示;
4. 输入“886”结束程序。
相关推荐
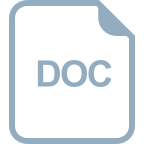
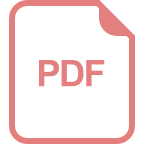









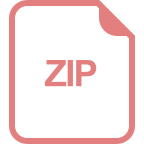
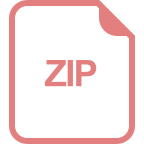
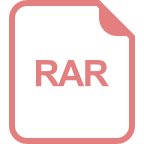