使用java完成上述问题
时间: 2024-02-25 11:56:53 浏览: 19
当然,使用 Java 也可以完成将二进制文件转换为 JSON 的操作。以下是一个简单的示例程序,假设二进制文件中的每个记录都是长度固定的,并且包含三个字段,分别是 ID、Name 和 Score。
```java
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONObject;
public class BinaryToJson {
public static void main(String[] args) throws IOException {
// 读取二进制文件
byte[] bytes = Files.readAllBytes(new File("students.bin").toPath());
// 解析二进制文件并将其转换为内存数据结构
List<Student> students = new ArrayList<>();
for (int i = 0; i < bytes.length; i += 30) {
String id = new String(bytes, i, 10, StandardCharsets.US_ASCII).trim();
String name = new String(bytes, i + 10, 10, StandardCharsets.US_ASCII).trim();
String score = new String(bytes, i + 20, 10, StandardCharsets.US_ASCII).trim();
students.add(new Student(id, name, score));
}
// 将内存数据结构转换为 JSON 格式
JSONArray jsonArray = new JSONArray();
for (Student student : students) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("id", student.getId());
jsonObject.put("name", student.getName());
jsonObject.put("score", student.getScore());
jsonArray.put(jsonObject);
}
String json = jsonArray.toString(4);
// 将 JSON 格式输出到文件
try (PrintWriter out = new PrintWriter("students.json")) {
out.println(json);
}
}
private static class Student {
private String id;
private String name;
private String score;
public Student(String id, String name, String score) {
this.id = id;
this.name = name;
this.score = score;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getScore() {
return score;
}
}
}
```
这个程序使用了 Java 的标准库,包括了文件读取和写入、字符串解析、JSON 库等功能。具体的操作步骤和 Python 版本类似,只是代码实现上有所不同。
相关推荐
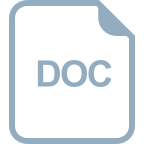
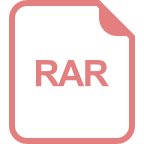
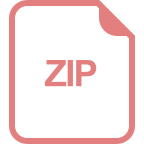














