C语言编写程序,计算两个复数的和、差、积、商。该结构体可以显示函数
时间: 2024-05-01 14:17:57 浏览: 155
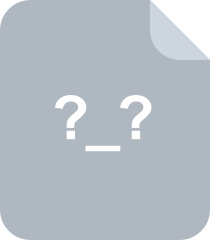
用C语言实现复数的运算
好的,以下是用C语言编写计算两个复数的和、差、积、商的程序:
```
#include <stdio.h>
struct complex {
float real;
float imag;
};
struct complex add(struct complex num1, struct complex num2) {
struct complex res;
res.real = num1.real + num2.real;
res.imag = num1.imag + num2.imag;
return res;
}
struct complex sub(struct complex num1, struct complex num2) {
struct complex res;
res.real = num1.real - num2.real;
res.imag = num1.imag - num2.imag;
return res;
}
struct complex mul(struct complex num1, struct complex num2) {
struct complex res;
res.real = (num1.real * num2.real) - (num1.imag * num2.imag);
res.imag = (num1.real * num2.imag) + (num1.imag * num2.real);
return res;
}
struct complex div(struct complex num1, struct complex num2) {
struct complex res;
float den = num2.real * num2.real + num2.imag * num2.imag;
res.real = (num1.real * num2.real + num1.imag * num2.imag) / den;
res.imag = (num1.imag * num2.real - num1.real * num2.imag) / den;
return res;
}
int main() {
struct complex num1, num2, res;
char op;
printf("Enter the first complex number (a + bi): ");
scanf("%f %f", &num1.real, &num1.imag);
printf("Enter the second complex number (a + bi): ");
scanf("%f %f", &num2.real, &num2.imag);
printf("Enter operation (+, -, *, /): ");
scanf(" %c", &op);
switch (op) {
case '+':
res = add(num1, num2);
printf("Result: %.2f + %.2fi\n", res.real, res.imag);
break;
case '-':
res = sub(num1, num2);
printf("Result: %.2f + %.2fi\n", res.real, res.imag);
break;
case '*':
res = mul(num1, num2);
printf("Result: %.2f + %.2fi\n", res.real, res.imag);
break;
case '/':
res = div(num1, num2);
printf("Result: %.2f + %.2fi\n", res.real, res.imag);
break;
default:
printf("Invalid operation\n");
}
return 0;
}
```
这个程序使用了结构体来存储复数,然后分别定义了四个函数来计算和、差、积、商。用户可以输入两个复数和一个运算符,然后程序根据运算符调用相应的函数来计算结果,并输出。
阅读全文
相关推荐
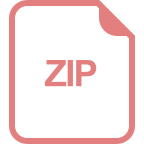















