otsu阈值分割算法实现
时间: 2023-10-10 15:13:21 浏览: 127
Otsu阈值分割算法是一种基于图像直方图的自适应阈值分割方法,它可以自动确定分割阈值,使得分割后的两个部分之间的类间方差最大,从而达到最佳分割效果。
以下是Python实现Otsu阈值分割算法的示例代码:
```python
import cv2
import numpy as np
def otsu_threshold(image):
# 计算图像直方图
hist, _ = np.histogram(image, bins=256)
# 归一化直方图
hist_norm = hist / float(image.size)
# 初始化变量
best_thresh = 0
best_var = 0
w0 = 0
u0 = 0
total_mean = np.mean(image)
# 遍历所有阈值
for thresh in range(256):
w1 = w0 + hist_norm[thresh]
if w1 == 0:
continue
u1 = (w0 * u0 + thresh * hist_norm[thresh]) / w1
u2 = (total_mean - w1 * u1) / (1 - w1)
var_between = w0 * (u0 - total_mean)**2 + w1 * (u1 - total_mean)**2
if var_between > best_var:
best_var = var_between
best_thresh = thresh
w0 = w1
u0 = u1
# 返回最佳阈值
return best_thresh
# 读取图像
image = cv2.imread('test.png', 0)
# 应用Otsu阈值分割算法
thresh = otsu_threshold(image)
binary_image = np.zeros_like(image)
binary_image[image > thresh] = 255
# 显示结果
cv2.imshow('Original Image', image)
cv2.imshow('Binary Image', binary_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`otsu_threshold`函数实现了Otsu阈值分割算法,输入为灰度图像,输出为最佳阈值。然后,我们可以根据最佳阈值将图像二值化,生成二值化图像。最后,使用OpenCV库的`imshow`函数显示原始图像和二值化图像。
阅读全文
相关推荐
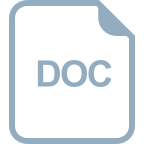
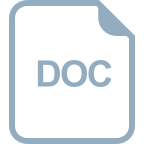
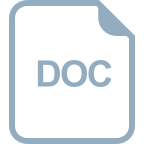











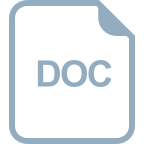



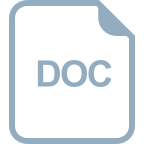