灰度直方图统计和灰度直方图均衡化都不使用已知函数完整代码
时间: 2024-02-25 18:54:30 浏览: 66
以下是使用Python实现灰度直方图统计和灰度直方图均衡化的完整代码:
```python
import numpy as np
import cv2
# 灰度直方图统计
def histogram(image):
height, width = image.shape[:2]
hist = np.zeros((256,))
for i in range(height):
for j in range(width):
pixel = image[i, j]
hist[pixel] += 1
return hist
# 灰度直方图均衡化
def equalize(image):
height, width = image.shape[:2]
cdf = np.zeros((256,))
hist = histogram(image)
sum = 0
map = np.zeros((256,), dtype=np.uint8)
for i in range(256):
sum += hist[i]
cdf[i] = sum / (height * width)
for i in range(256):
map[i] = np.uint8(round(cdf[i] * 255))
result = np.zeros((height, width), dtype=np.uint8)
for i in range(height):
for j in range(width):
result[i, j] = map[image[i, j]]
return result
# 读取图像
image = cv2.imread('lena.png')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 统计直方图
hist = histogram(gray)
# 绘制直方图
hist_img = np.zeros((256, 256), dtype=np.uint8)
for i in range(256):
cv2.line(hist_img, (i, 255), (i, 255-int(hist[i]/100)), 255)
# 直方图均衡化
eq = equalize(gray)
# 显示结果
cv2.imshow('original', gray)
cv2.imshow('histogram', hist_img)
cv2.imshow('equalized', eq)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`histogram`函数用于统计灰度直方图,`equalize`函数用于实现灰度直方图均衡化,`cv2.imread`函数用于读取图像,`cv2.cvtColor`函数用于将图像转换为灰度图,`cv2.line`函数用于绘制直方图,`cv2.imshow`函数用于显示图像。
阅读全文
相关推荐


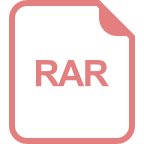
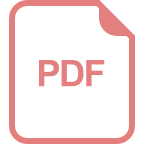
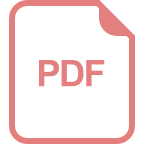
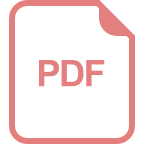
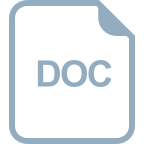
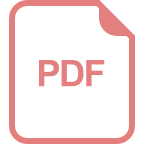
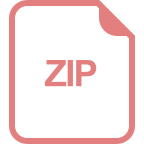
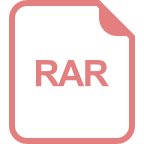
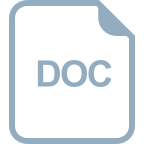
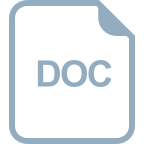
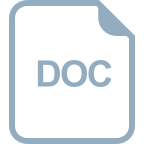
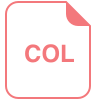
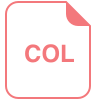

