eclipse如何连接SQLsevere数据库
时间: 2023-07-20 18:10:52 浏览: 48
要在Eclipse中连接SQL Server数据库,需要执行以下步骤:
1. 下载并安装Microsoft JDBC驱动程序。可以从Microsoft官方网站下载。
2. 在Eclipse中创建一个新的Java项目。
3. 在项目中创建一个新的类。
4. 在类中添加以下代码:
```
import java.sql.*;
public class SQLServerConnection {
public static void main(String[] args) {
// JDBC driver name and database URL
String JDBC_DRIVER = "com.microsoft.sqlserver.jdbc.SQLServerDriver";
String DB_URL = "jdbc:sqlserver://localhost:1433;databaseName=your_database_name";
// Database credentials
String USER = "your_database_username";
String PASS = "your_database_password";
Connection conn = null;
Statement stmt = null;
try{
// Register JDBC driver
Class.forName(JDBC_DRIVER);
// Open a connection
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
// Execute a query
System.out.println("Creating statement...");
stmt = conn.createStatement();
String sql;
sql = "SELECT id, name, age FROM your_table_name";
ResultSet rs = stmt.executeQuery(sql);
// Extract data from result set
while(rs.next()){
// Retrieve by column name
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
// Display values
System.out.print("ID: " + id);
System.out.print(", Name: " + name);
System.out.println(", Age: " + age);
}
// Clean-up environment
rs.close();
stmt.close();
conn.close();
}catch(SQLException se){
// Handle errors for JDBC
se.printStackTrace();
}catch(Exception e){
// Handle errors for Class.forName
e.printStackTrace();
}finally{
// Finally block used to close resources
try{
if(stmt!=null)
stmt.close();
}catch(SQLException se2){
}// nothing we can do
try{
if(conn!=null)
conn.close();
}catch(SQLException se){
se.printStackTrace();
}// end finally try
}// end try
System.out.println("Goodbye!");
}// end main
}// end SQLServerConnection
```
5. 在代码中替换以下内容:
- `JDBC_DRIVER`:将其设置为Microsoft JDBC驱动器的名称。
- `DB_URL`:将其设置为您的SQL Server数据库的URL。
- `USER`和`PASS`:将其替换为您的数据库用户名和密码。
- `your_database_name`:将其替换为您的数据库名称。
- `your_table_name`:将其替换为您要查询的表的名称。
6. 运行代码即可连接到SQL Server数据库并查询数据。
相关推荐
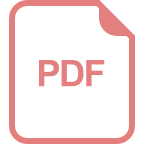
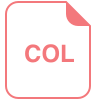













