java8写一个根据配置文件中的多重条件,对list中满足全部条件的数据进行skip处理的共通方法 点击复制后,将打开C知道体验页 以下是一个根据配置文件中的多重条件,对list中满足全部条件的数据进行skip处理的共通方法的示例代码:
时间: 2024-05-24 09:11:45 浏览: 140
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
public class SkipProcessor {
private Properties properties;
public SkipProcessor(String configFile) {
properties = new Properties();
try (InputStream input = new FileInputStream(configFile)) {
properties.load(input);
} catch (IOException e) {
e.printStackTrace();
}
}
public <T> List<T> skip(List<T> list) {
List<T> result = new ArrayList<>();
for (T t : list) {
if (shouldSkip(t)) {
continue;
}
result.add(t);
}
return result;
}
private <T> boolean shouldSkip(T t) {
for (String key : properties.stringPropertyNames()) {
String[] conditions = properties.getProperty(key).split(",");
boolean match = true;
for (String condition : conditions) {
String[] parts = condition.split("=");
String fieldName = parts[0];
String expectedValue = parts[1];
String actualValue = getFieldValue(t, fieldName);
if (!expectedValue.equals(actualValue)) {
match = false;
break;
}
}
if (match) {
return true;
}
}
return false;
}
private <T> String getFieldValue(T t, String fieldName) {
try {
return String.valueOf(t.getClass().getField(fieldName).get(t));
} catch (IllegalAccessException | NoSuchFieldException e) {
e.printStackTrace();
return null;
}
}
}
// 使用示例
class Person {
public String name;
public int age;
public String gender;
public Person(String name, int age, String gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", gender='" + gender + '\'' +
'}';
}
}
class Main {
public static void main(String[] args) {
List<Person> list = new ArrayList<>();
list.add(new Person("Alice", 25, "F"));
list.add(new Person("Bob", 30, "M"));
list.add(new Person("Charlie", 20, "M"));
list.add(new Person("David", 18, "M"));
list.add(new Person("Eve", 28, "F"));
SkipProcessor processor = new SkipProcessor("config.properties");
List<Person> result = processor.skip(list);
System.out.println(result);
}
}
// config.properties
# 跳过年龄小于20岁的男性
skip.condition.1=age<20,gender=M
阅读全文
相关推荐
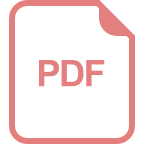
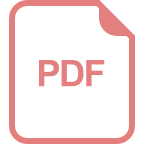
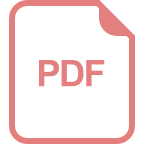














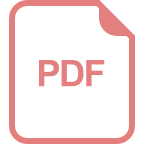
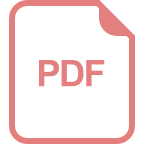