Warning: Synthesized away the following node(s): Warning: Synthesized away the following RAM node(s):
时间: 2024-05-20 11:11:07 浏览: 5
这个警告一般出现在 FPGA 设计中,意思是某些节点或者某些 RAM 被综合工具优化掉了,也就是在实际的硬件中不存在了。这可能会导致设计出现问题,需要检查一下是否对应的节点或者 RAM 确实不需要或者是否出现了错误。一些综合工具也提供了关闭综合优化的选项,可以尝试关闭这个选项,看看是否仍然会出现这个警告。
相关问题
Synthesized Classifiers for Zero-Shot Learning
Synthesized Classifiers for Zero-Shot Learning(零样本学习的合成分类器)是一种用于解决零样本学习问题的方法。在传统的分类任务中,我们通常有大量的标记样本来训练分类器。但在零样本学习中,我们面临的挑战是缺乏训练样本来学习未知类别的分类器。
为了解决这个问题,Synthesized Classifiers方法使用已有的类别信息和属性信息来合成新的分类器。具体而言,该方法首先通过提取类别的属性信息,例如物体的颜色、形状、纹理等,形成一个属性矩阵。然后,通过计算不同类别之间的属性相似度,构建一个属性相似度矩阵。接下来,使用这个属性相似度矩阵来合成新的分类器。
合成分类器的基本思想是根据已有类别的属性相似度来预测未知类别的属性,并根据预测的属性来进行分类。通过合成分类器,我们可以将未知类别映射到属性空间,并进行分类。
Synthesized Classifiers方法在零样本学习中具有较好的性能,并且可以应用于各种领域,例如图像分类、自然语言处理等。它为解决零样本学习问题提供了一种有效的方法。
synthesized.wav这里面是什么
`synthesized.wav` 是一个新的 WAV 文件,它是通过对原始 WAV 文件进行 LPC 分析,并使用得到的 LPC 系数合成得到的。合成后的信号被写入 `synthesized.wav` 文件中。这个新的 WAV 文件中的信号与原始 WAV 文件中的信号具有相同的采样率、声道数和采样位数,但是它的声音可能会有所不同,因为它是通过 LPC 合成得到的。
相关推荐
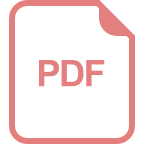
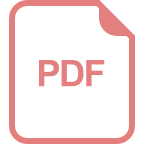
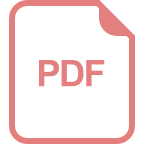












