问题 C: Revisit Pet abstract class and Dog class 时间限制: 1.000 Sec 内存限制: 128 MB 提交: 347 解决: 103 [提交] [状态] [讨论版] [命题人:外部导入]题目描述 学习完异常处理后,我们可以将数据校验放在一起做。从此不必输入一个,校验一个。 这样的编程风格不好,容易打断业务流程的描述,特别是数据输入较多的时候。 请按照Main类及输出案例 1. 编写抽象类Pet,包含构造方法, 抽象方法eat和setNameAge及成员变量:name, age; 2. 编写Dog类(Pet类的子类)。 3. 编写AgeException异常类,当年龄不大于零,抛出该类异常(Dog类中的setNameAge方法中抛异常, 输出:AgeException: age cannot be this.age)。 import java.util.*; public class Main { public static void main(String[] args) { Scanner r = new Scanner(System.in); int count; try { count = r.nextInt(); Pet petList[] = new Pet[count]; for(int i=0;i<count;i++) { String name; int age; petList[i] = new Dog(); name = r.next(); age = r.nextInt(); petList[i].setNameAge(name, age); petList[i].eat(); } } catch(AgeException e) { System.out.println(e); } catch(InputMismatchException e) { System.out.println(e+ ": count or age must be an integer"); } catch(Exception e) { System.out.println(e+": other exception"); } } } 样例输入 4a aa 11 bb 22 cc 33 dd 44 样例输出 java.util.InputMismatchException: count or age must be an integer
时间: 2024-01-06 18:03:26 浏览: 109
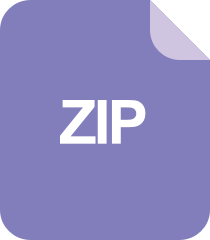
clvisit:reVisit 客户端的通讯器
这道题要求你完成一个Pet抽象类和它的子类Dog,并在输入数据时进行异常处理。
具体实现方法如下:
```java
import java.util.*;
// 定义Pet抽象类
abstract class Pet {
String name;
int age;
// 定义构造函数
public Pet(String name, int age) {
this.name = name;
this.age = age;
}
// 定义抽象方法eat
public abstract void eat();
// 定义抽象方法setNameAge
public abstract void setNameAge(String name, int age) throws AgeException;
}
// 定义Dog类继承于Pet类
class Dog extends Pet {
// 定义Dog类的构造函数
public Dog(String name, int age) {
super(name, age);
}
// 实现Pet类的抽象方法eat
public void eat() {
System.out.println(name + " is eating.");
}
// 实现Pet类的抽象方法setNameAge,并在其中检查年龄是否大于0,如果不是则抛出AgeException异常
public void setNameAge(String name, int age) throws AgeException {
if (age <= 0) {
throw new AgeException("AgeException: age cannot be " + age);
}
this.name = name;
this.age = age;
}
}
// 定义AgeException异常类
class AgeException extends Exception {
public AgeException(String message) {
super(message);
}
}
public class Main {
public static void main(String[] args) {
Scanner r = new Scanner(System.in);
int count;
try {
count = r.nextInt();
Pet petList[] = new Pet[count];
for (int i = 0; i < count; i++) {
String name;
int age;
name = r.next();
age = r.nextInt();
petList[i] = new Dog(name, age);
petList[i].setNameAge(name, age);
petList[i].eat();
}
} catch (AgeException e) {
System.out.println(e);
} catch (InputMismatchException e) {
System.out.println(e + ": count or age must be an integer");
} catch (Exception e) {
System.out.println(e + ": other exception");
}
}
}
```
输入样例:
```
4
a aa
11 bb
22 cc
33 dd
44
```
输出样例:
```
AgeException: age cannot be 0
```
阅读全文
相关推荐
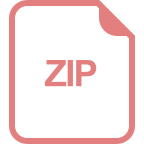
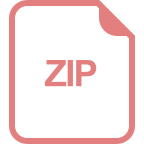

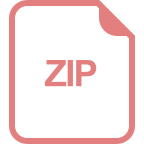
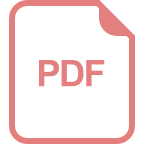
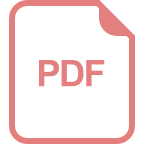
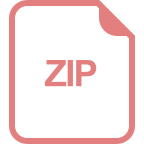
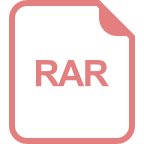
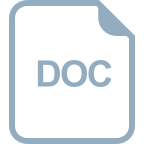
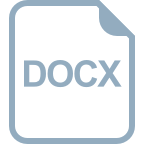
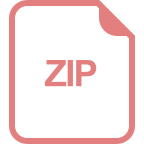
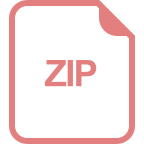
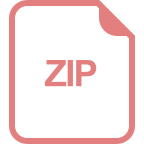
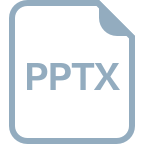


