Collectors.groupingBy获取一个属性出现最多的对象
时间: 2023-07-26 18:16:08 浏览: 84
可以使用Java 8中的Collectors.groupingBy方法和Collectors.counting方法来获取一个属性出现最多的对象。假设我们有一个对象列表,每个对象都有一个属性name,我们要获取出现最多的name对应的对象。可以使用以下代码:
```
Map<String, Long> nameCountMap = objectList.stream()
.collect(Collectors.groupingBy(Object::getName, Collectors.counting()));
String mostCommonName = Collections.max(nameCountMap.entrySet(), Map.Entry.comparingByValue()).getKey();
Object mostCommonObject = objectList.stream()
.filter(obj -> obj.getName().equals(mostCommonName))
.findFirst()
.orElse(null);
```
这段代码首先使用groupingBy方法对name进行分组,并使用counting方法计算每个name出现的次数,返回一个Map。然后使用Collections.max方法获取出现次数最多的name,再使用findFirst方法找到第一个name与最多出现次数的name相同的对象。
相关问题
collectors.groupingby多字段
`Collectors.groupingBy()`是Java Stream API中的一个实用工具方法,用于对数据进行分组处理,通常基于一个或多个键进行分类。当你有一个集合,想要按照两个或更多的属性值来组织元素时,可以使用这个方法。
例如,假设你有一个Person对象列表,每个Person都有name和age属性。你可以这样做:
```java
List<Person> people = ...; // 假设Person是一个类,有name和age属性
Map<tuple<String, Integer>, List<Person>> groupedPeople =
people.stream()
.collect(Collectors.groupingBy(Person::getNameAndAge)); // 使用方法引用,这里假定getNameAndAge是返回一个元组(name, age)的方法
// 现在groupedPeople是一个Map,key是元组形式的(name, age),value是具有相同(name, age)的所有Person对象组成的列表
```
在这里,`Person::getNameAndAge`是一个方法引用来获取每个Person对象的键值,通常是通过组合两个字段(如姓名和年龄)得到的。
Collectors.groupingBy(SiteDeviceRelatedDO::getSiteId, Collectors.mapping(e -> deviceMap.get(e.getDeviceId()), Collectors.toList()))把deviceMap中获取到的null加入到list集合中,出现All elements are null
`Collectors.groupingBy(SiteDeviceRelatedDO::getSiteId, Collectors.mapping(e -> deviceMap.get(e.getDeviceId()), Collectors.toList()))` 的作用是将一个元素为 `e` 的流按照 `SiteDeviceRelatedDO` 对象的 `siteId` 属性进行分组,对于每个分组,将其中的元素 `e` 转化为对应的 `Device` 对象,然后将这些 `Device` 对象放入一个 List 中。
如果 `deviceMap.get(e.getDeviceId())` 返回了 null,那么这个 null 也会被加入到 List 中。如果整个流中的元素在 `deviceMap` 中均没有对应的 `Device`,那么就会得到一个 Map,其中所有的 value 都是一个 List,其中所有元素都是 null。
如果你希望在每个 List 中去掉所有的 null 元素,可以在 `Collectors.toList()` 后再调用 `stream().filter(Objects::nonNull).collect(Collectors.toList())` 进行过滤,如下所示:
```
Map<Long, List<Device>> siteDeviceMap = eventList.stream()
.collect(Collectors.groupingBy(
SiteDeviceRelatedDO::getSiteId,
Collectors.mapping(e -> deviceMap.get(e.getDeviceId())
.stream()
.filter(Objects::nonNull)
.collect(Collectors.toList()),
Collectors.toList()
));
```
这里的 `eventList` 是一个元素为 `e` 的流,`deviceMap` 是一个从 `deviceId` 到 `Device` 的映射。首先,使用 `groupingBy` 操作将 `eventList` 中的元素按照 `SiteDeviceRelatedDO` 对象的 `siteId` 属性进行分组。然后,使用 `mapping` 操作将每个组中的元素 `e` 映射为对应的 `Device` 对象列表,如果 `deviceMap.get(e.getDeviceId())` 返回的是 null,那么这个 null 也会被映射到结果中。接着,使用 `filter` 操作过滤掉所有的 null 元素。最后,使用 `collect` 操作将过滤后的结果收集到一个 List 中,这个 List 中不会包含 null 元素。
阅读全文
相关推荐
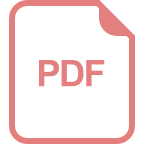
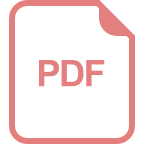
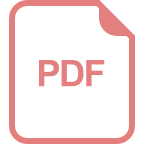




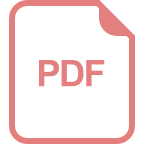








