public ReaderMenu(String readerId) { setBounds(100, 100, 478, 422); contentPane = new JPanel(); contentPane.setBorder(new EmptyBorder(5, 5, 5, 5)); setContentPane(contentPane); contentPane.setLayout(null); JButton btnNewButton = new JButton("图书查询"); btnNewButton.setFont(new Font("宋体", Font.BOLD, 15)); btnNewButton.setBounds(100,30,200,50); btnNewButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { BorrowingForm qf = new BorrowingForm(); qf.setVisible(true); } }); contentPane.add(btnNewButton); JButton btnNewButton_1 = new JButton("借阅记录"); btnNewButton_1.setBounds(100,100,200,50); btnNewButton_1.setFont(new Font("宋体", Font.BOLD, 15)); btnNewButton_1.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { ReadersBorrowingForm rbf=new ReadersBorrowingForm(readerId); rbf.setVisible(true); } }); JButton btnNewButton_2 = new JButton("退出"); btnNewButton_2.setBounds(100,170,200,50); btnNewButton_2.setFont(new Font("宋体", Font.BOLD, 15)); btnNewButton_2.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { new LogIn(); dispose(); } }); contentPane.add(btnNewButton_1); contentPane.add(btnNewButton_2); this.setSize(400,300); this.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); this.setResizable(false); this.setVisible(true); this.setLocationRelativeTo(null); } }
时间: 2024-04-01 20:32:31 浏览: 14
这是一个Java类,名为ReaderMenu,它继承自JFrame类。构造函数接受一个String类型的readerId参数。
该类创建了一个JPanel作为容器,设置了边界和布局,并添加了三个按钮:
1. "图书查询"按钮:创建了一个BorrowingForm对象并将其设置为可见。
2. "借阅记录"按钮:创建了一个ReadersBorrowingForm对象,并将读者ID作为参数传递给它,然后将其设置为可见。
3. "退出"按钮:创建了一个新的LogIn对象并关闭当前窗口。
该类还设置了窗口的大小、关闭方式、是否可调整大小、是否可见以及位置居中。
相关问题
public class BFormService { Book book=new Book(); BFormdao dao=new BFormdao(); public List<Book> select_by_id(Book bf) { List<Book> aList=dao.select_by_id(bf); return aList; } public List<Book> select_by_isbn(Book bf) { List<Book> aList=dao.select_by_isbn(bf); return aList; } public List<BForm> selectByBookNameAndReaderId(String BookName,String ReaderId){ List<BForm> bList=dao.selectByBookNameAndReaderId(BookName,ReaderId); return bList; } public List<BForm> selectByISBNAndReaderId(String ISBN,String ReaderId){ List<BForm> bList=dao.selectByISBNAndReaderId(ISBN,ReaderId); return bList; } }
这是一个Java类,名为BFormService。它包含了一个Book对象和一个BFormdao对象,并提供了四个方法:
1. select_by_id(Book bf):根据图书ID查询图书信息并返回一个Book类型的List集合。
2. select_by_isbn(Book bf):根据图书ISBN查询图书信息并返回一个Book类型的List集合。
3. selectByBookNameAndReaderId(String BookName,String ReaderId):根据图书名称和读者ID查询借阅信息并返回一个BForm类型的List集合。
4. selectByISBNAndReaderId(String ISBN,String ReaderId):根据图书ISBN和读者ID查询借阅信息并返回一个BForm类型的List集合。
public Integer getReaderId() { return this.readerId; } public void setReaderId(Integer readerId) { this.readerId = readerId; } public String getReaderName() { return this.readerName; } public void setReaderName(String readerName) { this.readerName = readerName; }
这是一个Java类中的部分代码,其中定义了四个方法,用于获取或设置阅读者的ID和姓名属性。
在Java中,通常将对象的属性设置为私有的,并提供公有的getter和setter方法,以便外部程序可以访问和修改这些属性。在该示例中,定义了两个名为 `readerId` 和 `readerName` 的私有属性,并分别提供了名为 `getReaderId` 和 `setReaderId`、`getReaderName` 和 `setReaderName` 的getter和setter方法。
这些方法的作用如下:
- `getReaderId` 方法用于获取阅读者的ID属性值,并将其返回给调用者。
- `setReaderId` 方法用于设置阅读者的ID属性值,并将其保存到对象中。
- `getReaderName` 方法用于获取阅读者的姓名属性值,并将其返回给调用者。
- `setReaderName` 方法用于设置阅读者的姓名属性值,并将其保存到对象中。
在使用该Java类时,可以通过调用这些getter和setter方法,访问或修改阅读者的ID和姓名属性值,例如:
```java
Reader reader = new Reader();
reader.setReaderId(1001);
reader.setReaderName("Tom");
System.out.println(reader.getReaderId()); // 输出1001
System.out.println(reader.getReaderName()); // 输出Tom
```
相关推荐
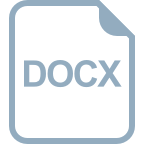
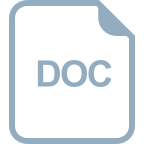
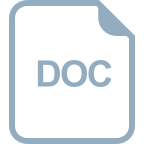












