osgearth3.2封装成C#可调用的DLL的实现代码
时间: 2024-02-15 17:03:31 浏览: 207
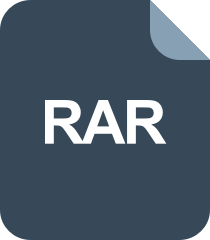
bartender 10.0 C#动态调用DLL
将 OSGEarth 3.2 封装成 C# 可调用的 DLL 的实现代码示例如下:
1. 创建一个 C++ 动态链接库项目,并将 OSGEarth 的头文件和库文件引入。在 Visual Studio 中,可以通过 `项目属性 -> C/C++ -> 常规 -> 附加包含目录` 和 `项目属性 -> 链接器 -> 常规 -> 附加库目录` 分别设置头文件和库文件的路径。
2. 在 C++ 项目中编写需要封装的函数,并将它们导出为 DLL。例如,我们需要将 OSGEarth 中的 `osg::Vec3d` 类型转换为 C# 中的 `System.Windows.Media.Media3D.Point3D` 类型:
```cpp
#include <osg/Vec3d>
extern "C" __declspec(dllexport) void Vec3dToPoint3D(const osg::Vec3d& vec, System::Windows::Media::Media3D::Point3D& point)
{
point.X = vec.x();
point.Y = vec.y();
point.Z = vec.z();
}
```
3. 在 C# 项目中引入 C++ DLL,并使用 `DllImport` 属性导入 C++ 的函数。例如,在 C# 项目中调用上述函数:
```csharp
using System.Runtime.InteropServices;
using System.Windows.Media.Media3D;
class Program
{
[DllImport("OSGEarthInterop.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern void Vec3dToPoint3D(ref Vector3d vec, out Point3D point);
static void Main(string[] args)
{
Vector3d vec = new Vector3d(1.0, 2.0, 3.0);
Point3D point;
Vec3dToPoint3D(ref vec, out point);
Console.WriteLine(point.ToString()); // 输出 (1, 2, 3)
}
}
```
4. 编译 C++ 和 C# 项目,并将 C++ DLL 拷贝到 C# 项目的输出目录中。在运行 C# 项目时,C++ DLL 会被自动加载并使用。
需要注意的是,在跨语言调用时,需要确保数据类型的一致性和内存对齐方式的一致性。在上述例子中,我们使用了 `__declspec(dllexport)` 和 `CallingConvention = CallingConvention.Cdecl` 来确保函数的导出和调用方式的一致性。
阅读全文
相关推荐
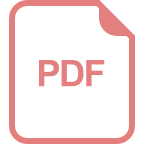
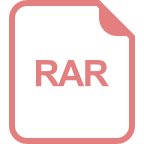















