使用springboot ,查询数据库的某一列数据,该列数据每一行都是一个数组,现在我想将查询出来的数组与数组[0,1,2,3,4,5,6,7,89,]做内积,给出具体代码解释和需要配置的地方
时间: 2024-05-15 13:14:32 浏览: 7
假设你的数据表名为`table_name`,要查询的列名为`array_column`,内积的数组为`[0,1,2,3,4,5,6,7,89]`,你可以使用如下代码:
```java
import org.springframework.jdbc.core.JdbcTemplate;
import java.util.List;
public class ExampleClass {
private final JdbcTemplate jdbcTemplate;
public ExampleClass(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
public void innerProduct() {
List<String> arrayColumnList = jdbcTemplate.queryForList("SELECT array_column FROM table_name", String.class);
int[] innerProductArray = new int[arrayColumnList.size()];
int[] innerProductVector = {0, 1, 2, 3, 4, 5, 6, 7, 89};
int index = 0;
for (String arrayColumn : arrayColumnList) {
String[] array = arrayColumn.replaceAll("[\\[\\]]", "").split(",");
int sum = 0;
for (int i = 0; i < array.length; i++) {
sum += Integer.parseInt(array[i].trim()) * innerProductVector[i];
}
innerProductArray[index++] = sum;
}
// do something with the innerProductArray
}
}
```
解释:
1. 首先通过`jdbcTemplate.queryForList`方法查询出所有的`array_column`值,返回的是一个`List<String>`。
2. 遍历`arrayColumnList`中的每一个元素,对其进行内积的计算。内积的计算方法是将该列值解析成一个整数数组,然后与`innerProductVector`相乘累加得到内积。
3. 将每个结果保存到`innerProductArray`数组中。
需要配置的地方是`JdbcTemplate`的配置,你需要在`application.properties`或`application.yml`文件中加入数据库连接信息,例如:
```yaml
spring:
datasource:
url: jdbc:mysql://localhost:3306/db_name
username: username
password: password
driver-class-name: com.mysql.jdbc.Driver
```
同时,在Spring Boot的配置类中注入`JdbcTemplate`,例如:
```java
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.core.JdbcTemplate;
import javax.sql.DataSource;
@Configuration
public class ExampleConfig {
@Bean
public DataSource dataSource() {
return DataSourceBuilder.create()
.url("jdbc:mysql://localhost:3306/db_name")
.username("username")
.password("password")
.driverClassName("com.mysql.jdbc.Driver")
.build();
}
@Bean
public JdbcTemplate jdbcTemplate(DataSource dataSource) {
return new JdbcTemplate(dataSource);
}
}
```
其中,`dataSource()`方法返回一个`DataSource`对象,`jdbcTemplate()`方法注入了前面创建的`DataSource`对象,并返回一个`JdbcTemplate`对象。这样,在`ExampleClass`中就可以通过构造函数注入`JdbcTemplate`了。
相关推荐
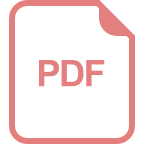
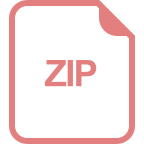














