使用java的springboot,写一个将数据库某一列的值都与[1,2,3,4,5,6,7,8,9]这个数组做内积
时间: 2024-05-04 18:21:51 浏览: 116
假设要将数据库中表table的某一列column与数组[1, 2, 3, 4, 5, 6, 7, 8, 9]做内积,可以按照以下步骤实现:
1. 首先,在Spring Boot项目中,需要引入对数据库的依赖,例如使用Spring Data JPA来操作数据库:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
```
2. 接着,定义一个JPA实体类,对应数据库中的表结构,例如:
```java
@Entity
@Table(name = "table")
public class TableEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String column;
// getters and setters
}
```
3. 在Spring Boot应用程序中,定义一个服务类,用于查询数据库中的数据,并将查询结果与数组[1, 2, 3, 4, 5, 6, 7, 8, 9]做内积,例如:
```java
@Service
public class TableService {
@Autowired
private TableRepository tableRepository;
public void calculateInnerProduct() {
// 查询数据库中的所有数据
List<TableEntity> list = tableRepository.findAll();
// 遍历查询结果,将每行数据的column字段与数组[1, 2, 3, 4, 5, 6, 7, 8, 9]做内积
for (TableEntity entity : list) {
String column = entity.getColumn();
int[] array = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int innerProduct = 0;
for (int i = 0; i < array.length; i++) {
innerProduct += Integer.parseInt(String.valueOf(column.charAt(i))) * array[i];
}
System.out.println("Inner product of " + column + " is " + innerProduct);
}
}
}
```
4. 最后,定义一个JpaRepository接口,用于操作数据库中的数据,例如:
```java
@Repository
public interface TableRepository extends JpaRepository<TableEntity, Long> {
}
```
这样,在Spring Boot应用程序中,就可以通过调用TableService的calculateInnerProduct方法来将数据库中某一列的值都与数组[1, 2, 3, 4, 5, 6, 7, 8, 9]做内积了。
阅读全文
相关推荐
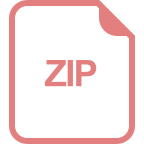
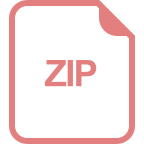
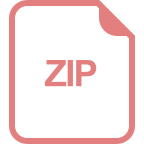
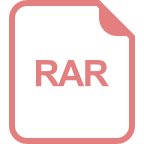
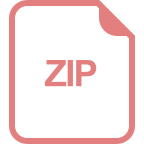
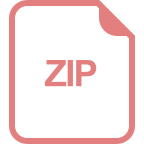
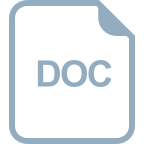
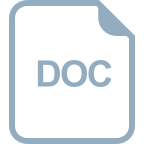
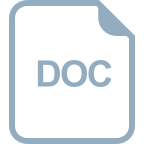
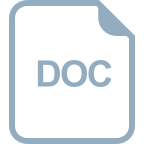
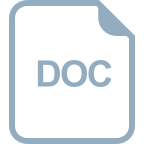
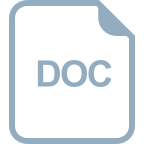
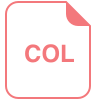




