c++实现万年历,用户只用输入年份,就可以输出这一年的年历。要求:利用循环方法产生年历数据,使用数据缓冲区存储和输出,将缓冲区看作一个二维平面,设计年历坐标系 ,按坐标填入星期表头,计算每天的坐标,填入日期内容,最后一起输出。 将一个月作为一个子程序,循环调用月内按天循环,事先存储每个月的天数
时间: 2023-10-16 13:09:01 浏览: 39
以下是一个简单的 C++ 实现万年历的代码,具体实现方式可能有所不同,但基本思路是相似的:
```cpp
#include <iostream>
using namespace std;
// 存储每个月的天数
const int monthDays[12] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
// 判断是否为闰年
bool isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return true;
}
return false;
}
// 计算某一年某一月的天数
int getDaysOfMonth(int year, int month) {
int days = monthDays[month - 1];
if (month == 2 && isLeapYear(year)) {
days++;
}
return days;
}
// 输出某一月的日历
void printMonth(int year, int month) {
// 计算该月第一天是星期几
int weekDay = (year + year / 4 - year / 100 + year / 400 + (13 * month + 8) / 5 + 1) % 7;
// 输出表头
char weekDays[7][4] = {"Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"};
for (int i = 0; i < 7; i++) {
cout << weekDays[i] << "\t";
}
cout << endl;
// 输出日期
int days = getDaysOfMonth(year, month);
int day = 1;
int row = 0;
int col = weekDay;
int calendar[6][7] = {0};
while (day <= days) {
calendar[row][col] = day++;
col++;
if (col == 7) {
row++;
col = 0;
}
}
// 输出日历
for (int i = 0; i < 6; i++) {
for (int j = 0; j < 7; j++) {
if (calendar[i][j] == 0) {
cout << "\t";
} else {
printf("%2d\t", calendar[i][j]);
}
}
cout << endl;
}
}
// 输出整年的日历
void printYear(int year) {
for (int i = 1; i <= 12; i++) {
cout << "-----------------------" << endl;
cout << " " << i << "月" << endl;
cout << "-----------------------" << endl;
printMonth(year, i);
}
}
int main() {
int year;
cout << "请输入年份:";
cin >> year;
printYear(year);
return 0;
}
```
该代码通过计算某一年某一月的第一天是星期几,然后按照星期和日期的坐标填充到一个缓冲区中,最后输出整个日历。如果需要输出到文件或其他设备,可以在代码中做相应的修改。
相关推荐
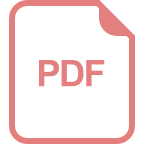
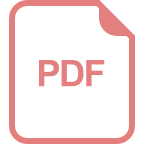














