android zipoutputstream 使用
时间: 2024-05-01 09:23:09 浏览: 110
ZipOutputStream 是一个 Java 类,可以用于创建压缩文件。在 Android 上,您可以使用它来创建和压缩文件。
下面是一个简单的示例代码,演示如何使用 ZipOutputStream 来创建一个压缩文件:
```java
public static void createZipFile(String sourceFolderPath, String outputFilePath) throws IOException {
File sourceFolder = new File(sourceFolderPath);
FileOutputStream fos = new FileOutputStream(outputFilePath);
ZipOutputStream zos = new ZipOutputStream(fos);
addFolderToZip(sourceFolder, zos, sourceFolder.getName());
zos.close();
fos.close();
}
private static void addFolderToZip(File folder, ZipOutputStream zip, String baseName) throws IOException {
File[] files = folder.listFiles();
for (File file : files) {
if (file.isDirectory()) {
addFolderToZip(file, zip, baseName + "/" + file.getName());
} else {
FileInputStream fis = new FileInputStream(file);
ZipEntry zipEntry = new ZipEntry(baseName + "/" + file.getName());
zip.putNextEntry(zipEntry);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) > 0) {
zip.write(buffer, 0, len);
}
fis.close();
zip.closeEntry();
}
}
}
```
在上面的代码中,createZipFile 方法接受源文件夹路径和输出文件路径作为参数。它会递归遍历文件夹中的所有文件和子文件夹,并将它们添加到 ZipOutputStream 中。addFolderToZip 方法用于递归添加文件夹和文件。它使用 FileInputStream 来读取文件内容,并将其添加到 ZipOutputStream 中。最后,ZipOutputStream 和 FileOutputStream 都会被关闭。
您可以使用以下代码调用 createZipFile 方法:
```java
try {
createZipFile("/path/to/source/folder", "/path/to/output/file.zip");
} catch (IOException e) {
e.printStackTrace();
}
```
请注意,您需要在 AndroidManifest.xml 文件中声明 WRITE_EXTERNAL_STORAGE 权限才能创建压缩文件。
阅读全文
相关推荐
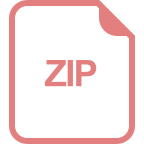
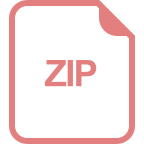
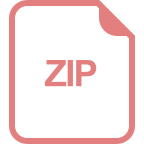
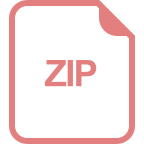
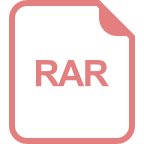
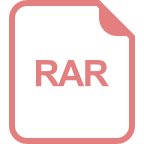
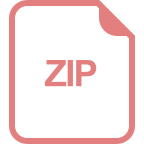
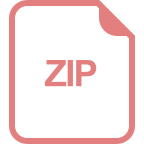
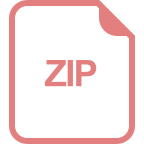
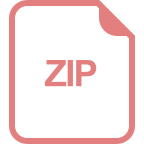
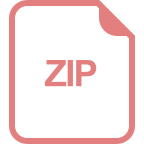
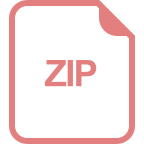
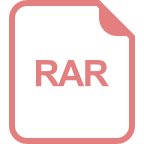
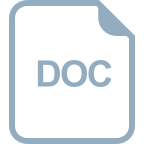


