class Student {public: Student(int n, string nam ) {num=n; name=nam; } void display() {cout<<"num:"<<num<<endl; cout<<"name:"<<name<<endl; } protected: int num; string name; }; class Student1: public Student {public: ▁▁▁(3分) {age=a; } void show( ) {display(); cout<<"age: "<<age<<endl; } private: int age; }; class Student2:public Student1 {public: ▁▁▁(3分) {score=s;} void show_all() {show(); cout<<"score:"<<score<<endl; } private: int score; };
时间: 2024-03-07 11:52:40 浏览: 21
在 class Student1 中,应该定义一个带参数的构造函数,将 age 初始化为给定的值。应该写成:`Student1(int n, string nam, int a): Student(n, nam), age(a) {}`。
在 class Student2 中,应该定义一个带参数的构造函数,将 score 初始化为给定的值。应该写成:`Student2(int n, string nam, int a, int s): Student1(n, nam, a), score(s) {}`。
相关问题
#include <iostream> #include<string> using namespace std; class Student {public: Student(int n, string nam ) {num=n; name=nam; } void display() {cout<<"num:"<<num<<endl; cout<<"name:"<<name<<endl; } protected: int num; string name; }; class Student1: public Student {public: 空缺 {age=a; } void show( ) {display(); cout<<"age: "<<age<<endl; } private: int age; }; class Student2:public Student1 {public: 空缺 {score=s;} void show_all() {show(); cout<<"score:"<<score<<endl; } private: int score; }; int main( ) {Student2 stud(10010,"Li",17,89); stud.show_all( ); 补全 return 0; }
在主函数中,需要补全一个输出语句,以便输出 stud 对象的信息。
可以在 stud.show_all() 函数调用之后,加上一行代码:
```
cout << "Student2 object information:" << endl;
```
这样就可以在输出学生的信息之前,先输出一条描述信息,以便更好地区分输出内容。完整的代码如下:
```
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
Student(int n, string nam) {
num = n; name = nam;
}
void display() {
cout << "num:" << num << endl;
cout << "name:" << name << endl;
}
protected:
int num;
string name;
};
class Student1 : public Student {
public:
Student1(int n, string nam, int a) : Student(n, nam) {
age = a;
}
void show() {
display();
cout << "age: " << age << endl;
}
private:
int age;
};
class Student2 : public Student1 {
public:
Student2(int n, string nam, int a, int s) : Student1(n, nam, a) {
score = s;
}
void show_all() {
show();
cout << "score: " << score << endl;
}
private:
int score;
};
int main() {
Student2 stud(10010, "Li", 17, 89);
cout << "Student2 object information:" << endl;
stud.show_all();
return 0;
}
```
执行程序后,输出结果如下:
```
Student2 object information:
num:10010
name:Li
age: 17
score: 89
```
#include<iostream> #include<string> using namespace std; class Student { public: Student(int n,string nam) { num=n; name=nam; } void display() { cout<<"num:"<<num<<endl; cout<<"name:"<<name<<endl; } protected: int num; string name; }; class Student1:public Student { public: Student1(int n,string name,int a):Student(n,nam) { age=a; } void show() { display(); cout<<"age:"<<age<<endl; } private: int age; }; class Student2:public Student1 { public: Student2(int n,string name,int a,int s):Student1(n,nam,a) { sex=s; } void show_all() { show(); cout<<"score:"<<score<<endl; } private: int score; }; int main() { Student2 stud(10010,"zaiweitao",17,89); stud.show_all(); return 0; }哪里错了?
您在派生类 `Student1` 的构造函数中将 `nam` 作为参数传递,但 `nam` 并没有定义,应该改为 `name`,即:
```
Student1(int n, string name, int a) : Student(n, name) {
age = a;
}
```
同样,在派生类 `Student2` 的构造函数中,应该将参数 `name` 改为 `nam` 或 `name`,并且要在构造函数中初始化 `score`,否则会有编译错误。改正后的代码如下:
```
class Student1 : public Student {
public:
Student1(int n, string name, int a) : Student(n, name) {
age = a;
}
void show() {
display();
cout << "age:" << age << endl;
}
private:
int age;
};
class Student2 : public Student1 {
public:
Student2(int n, string name, int a, int s) : Student1(n, name, a) {
score = s;
}
void show_all() {
show();
cout << "score:" << score << endl;
}
private:
int score;
};
int main() {
Student2 stud(10010, "zaiweitao", 17, 89);
stud.show_all();
return 0;
}
```
注意:在使用继承时,每个派生类的构造函数必须调用直接基类的构造函数,以确保直接基类的成员得到初始化。
相关推荐
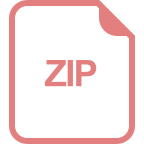












