java利用网页布局做一个投票小程序
时间: 2023-07-28 22:10:06 浏览: 117
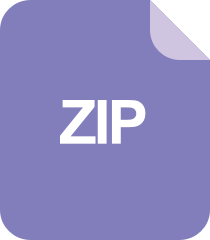
基于JAVA开发的(选课投票)小程序
要做一个投票小程序,可以利用 Java 和 HTML/CSS/JavaScript 来实现。以下是一个简单的示例:
1. 首先,创建一个 HTML 页面,包含投票选项和投票按钮。例如:
```
<!DOCTYPE html>
<html>
<head>
<title>投票小程序</title>
</head>
<body>
<h1>请选择你喜欢的编程语言:</h1>
<form>
<input type="radio" name="vote" value="java"> Java<br>
<input type="radio" name="vote" value="python"> Python<br>
<input type="radio" name="vote" value="javascript"> JavaScript<br>
<input type="submit" value="投票">
</form>
</body>
</html>
```
2. 在 Java 中编写一个简单的 HTTP 服务器,用来处理用户提交的投票信息。例如:
```
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetSocketAddress;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpServer;
public class VoteServer {
public static void main(String[] args) throws Exception {
HttpServer server = HttpServer.create(new InetSocketAddress(8000), 0);
server.createContext("/vote", new VoteHandler());
server.setExecutor(null);
server.start();
System.out.println("服务器已启动");
}
static class VoteHandler implements HttpHandler {
public void handle(HttpExchange t) throws IOException {
String response = "感谢您的投票!";
String query = t.getRequestURI().getQuery();
if (query != null && query.contains("vote=")) {
String vote = query.split("=")[1];
// TODO: 将投票信息保存到数据库中
}
t.sendResponseHeaders(200, response.length());
OutputStream os = t.getResponseBody();
os.write(response.getBytes());
os.close();
}
}
}
```
3. 在 HTML 页面中添加 JavaScript 代码,用来向服务器发送投票请求。例如:
```
<script>
const form = document.querySelector('form');
form.addEventListener('submit', (event) => {
event.preventDefault();
const vote = document.querySelector('input[name="vote"]:checked').value;
const xhr = new XMLHttpRequest();
xhr.open('GET', `/vote?vote=${vote}`);
xhr.onload = () => {
if (xhr.status === 200) {
alert(xhr.responseText);
} else {
alert('投票失败');
}
};
xhr.send();
});
</script>
```
这样,用户在页面中选择一个编程语言并点击投票按钮后,JavaScript 代码会向服务器发送一个 GET 请求,服务器会处理请求并保存投票信息。最后,页面会弹出一个感谢投票的提示框。
阅读全文
相关推荐
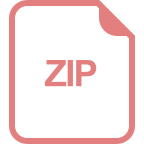
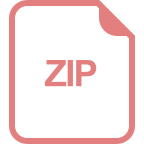















