用python写一段基于GAN的图像去雾代码
时间: 2024-02-18 12:59:38 浏览: 42
好的,以下是一段基于GAN的图像去雾代码,使用的是Python语言和TensorFlow框架。需要注意的是,这段代码仅供参考,实际效果可能需要根据具体情况调整。
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, Conv2D, MaxPooling2D, UpSampling2D, Flatten, Reshape, BatchNormalization, LeakyReLU
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.datasets import cifar10
import numpy as np
import matplotlib.pyplot as plt
# 加载数据
(x_train, _), (x_test, _) = cifar10.load_data()
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
# 添加噪声,模拟雾化效果
noise_factor = 0.3
x_train_noisy = x_train + noise_factor * np.random.normal(loc=0.0, scale=1.0, size=x_train.shape)
x_test_noisy = x_test + noise_factor * np.random.normal(loc=0.0, scale=1.0, size=x_test.shape)
x_train_noisy = np.clip(x_train_noisy, 0., 1.)
x_test_noisy = np.clip(x_test_noisy, 0., 1.)
# 定义生成器
def generator():
input_layer = Input(shape=(32, 32, 3))
x = Conv2D(32, (3, 3), padding='same')(input_layer)
x = BatchNormalization()(x)
x = LeakyReLU()(x)
x = Conv2D(64, (3, 3), padding='same')(x)
x = BatchNormalization()(x)
x = LeakyReLU()(x)
x = Conv2D(128, (3, 3), padding='same')(x)
x = BatchNormalization()(x)
x = LeakyReLU()(x)
x = Conv2D(256, (3, 3), padding='same')(x)
x = BatchNormalization()(x)
x = LeakyReLU()(x)
x = Conv2D(3, (3, 3), padding='same', activation='tanh')(x)
output_layer = x
model = Model(input_layer, output_layer)
return model
# 定义判别器
def discriminator():
input_layer = Input(shape=(32, 32, 3))
x = Conv2D(32, (3, 3), padding='same')(input_layer)
x = BatchNormalization()(x)
x = LeakyReLU()(x)
x = MaxPooling2D()(x)
x = Conv2D(64, (3, 3), padding='same')(x)
x = BatchNormalization()(x)
x = LeakyReLU()(x)
x = MaxPooling2D()(x)
x = Conv2D(128, (3, 3), padding='same')(x)
x = BatchNormalization()(x)
x = LeakyReLU()(x)
x = MaxPooling2D()(x)
x = Flatten()(x)
x = Dense(1, activation='sigmoid')(x)
output_layer = x
model = Model(input_layer, output_layer)
return model
# 定义GAN模型
def gan(generator, discriminator):
discriminator.trainable = False
input_layer = Input(shape=(32, 32, 3))
x = generator(input_layer)
output_layer = discriminator(x)
model = Model(input_layer, output_layer)
return model
# 编译模型
gen = generator()
dis = discriminator()
gan = gan(gen, dis)
gen_opt = Adam(lr=0.0002, beta_1=0.5)
dis_opt = Adam(lr=0.0002, beta_1=0.5)
gen.compile(loss='binary_crossentropy', optimizer=gen_opt)
dis.compile(loss='binary_crossentropy', optimizer=dis_opt)
gan.compile(loss='binary_crossentropy', optimizer=gen_opt)
# 训练模型
epochs = 100
batch_size = 128
for epoch in range(epochs):
for i in range(x_train_noisy.shape[0] // batch_size):
real_images = x_train_noisy[i * batch_size:(i + 1) * batch_size]
real_labels = np.ones((batch_size, 1))
fake_images = gen.predict(real_images)
fake_labels = np.zeros((batch_size, 1))
dis_loss_real = dis.train_on_batch(real_images, real_labels)
dis_loss_fake = dis.train_on_batch(fake_images, fake_labels)
dis_loss = 0.5 * np.add(dis_loss_real, dis_loss_fake)
gen_loss = gan.train_on_batch(real_images, real_labels)
print('Epoch %d: dis_loss=%.4f, gen_loss=%.4f' % (epoch + 1, dis_loss, gen_loss))
# 预测并可视化结果
decoded_imgs = gen.predict(x_test_noisy)
n = 10
plt.figure(figsize=(20, 4))
for i in range(n):
# 原始图像
ax = plt.subplot(3, n, i + 1)
plt.imshow(x_test_noisy[i])
plt.title('Noisy')
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
# 去雾后的图像
ax = plt.subplot(3, n, i + 1 + n)
plt.imshow(decoded_imgs[i])
plt.title('De-noised')
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
# 原始图像
ax = plt.subplot(3, n, i + 1 + n * 2)
plt.imshow(x_test[i])
plt.title('Original')
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
plt.show()
```
以上代码使用了一个简单的生成器和判别器,训练了100轮,然后对测试集数据进行了去雾处理。最后,将原始图像、去雾后的图像和有噪声的图像进行对比展示,以便评估去雾效果。
相关推荐
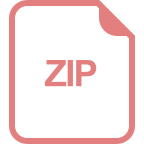














