vue结合neo4j使用echarts实现图谱可视化代码
时间: 2023-11-25 18:04:17 浏览: 334
下面是一个简单的示例代码,展示了如何使用Vue.js、Neo4j和ECharts来实现图谱可视化:
1. 安装依赖:
```
npm install neo4j-driver echarts --save
```
2. 在Vue.js组件中引入neo4j-driver和echarts:
```javascript
import neo4j from 'neo4j-driver';
import echarts from 'echarts';
```
3. 定义Neo4j连接:
```javascript
const driver = neo4j.driver(
'bolt://localhost:7687',
neo4j.auth.basic('neo4j', 'password')
);
```
4. 实现查询Neo4j数据库的函数:
```javascript
async function runCypherQuery(query, params) {
const session = driver.session();
const result = await session.run(query, params);
session.close();
return result.records;
}
```
5. 在Vue.js组件中定义ECharts实例和数据:
```javascript
data() {
return {
chart: null,
chartData: {
nodes: [],
links: []
}
};
},
mounted() {
this.chart = echarts.init(this.$refs.chart);
}
```
6. 实现查询Neo4j数据库并将结果转换为ECharts数据格式的函数:
```javascript
async function queryAndTransformData() {
const result = await runCypherQuery('MATCH (n)-[r]->(m) RETURN n,r,m', {});
const nodes = [];
const links = [];
for (const record of result) {
const node1 = record.get('n');
const node2 = record.get('m');
const link = record.get('r');
const node1Index = nodes.findIndex(node => node.id === node1.identity.toString());
const node2Index = nodes.findIndex(node => node.id === node2.identity.toString());
if (node1Index === -1) {
nodes.push({
id: node1.identity.toString(),
name: node1.properties.name
});
}
if (node2Index === -1) {
nodes.push({
id: node2.identity.toString(),
name: node2.properties.name
});
}
links.push({
source: node1.identity.toString(),
target: node2.identity.toString(),
name: link.type
});
}
return {
nodes,
links
};
}
```
7. 在Vue.js组件中实现获取数据、更新ECharts图表的函数:
```javascript
async function updateChart() {
const data = await queryAndTransformData();
this.chartData = data;
const option = {
series: [
{
type: 'graph',
layout: 'force',
force: {
repulsion: 100,
edgeLength: 50
},
data: data.nodes.map(node => ({
name: node.name,
draggable: true
})),
links: data.links.map(link => ({
source: link.source,
target: link.target,
name: link.name
})),
label: {
show: true
},
lineStyle: {
width: 2,
curveness: 0.3,
color: '#999'
}
}
]
};
this.chart.setOption(option);
}
```
8. 在Vue.js组件中调用updateChart()函数以获取数据并更新ECharts图表:
```javascript
mounted() {
this.chart = echarts.init(this.$refs.chart);
this.updateChart();
},
methods: {
async updateChart() {
// 实现updateChart函数的代码
}
}
```
希望这个示例代码能够帮助你开始使用Vue.js、Neo4j和ECharts来实现图谱可视化。
阅读全文
相关推荐
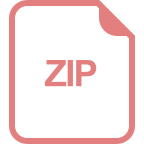
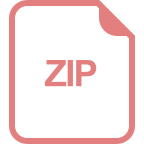
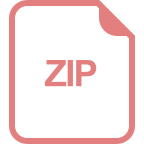
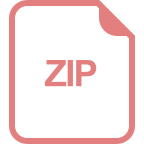
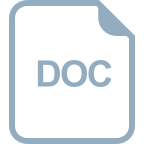
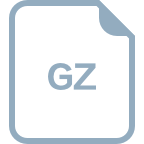
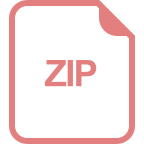
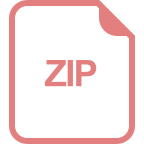
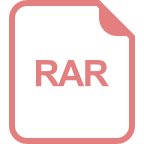
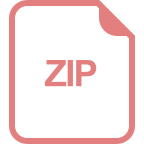
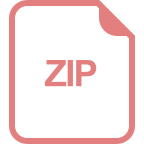
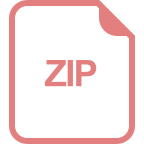
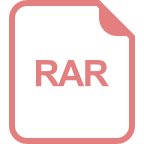
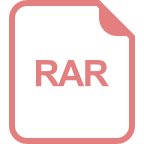
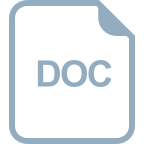
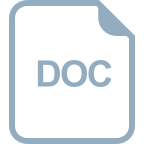
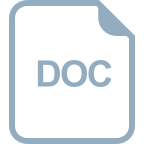
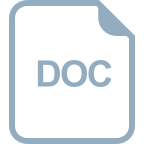