python写代码 编写函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以字典的形式返回结果。 例如 输入s: ”Hello World!778@#$Welcome!” 输出: {'upper': 2, 'lower': 16, 'digit': 3, 'other': 6}
时间: 2023-05-26 09:04:54 浏览: 33
输出d: {'upper': 2, 'lower': 8, 'digit': 3, 'other': 2}
这个函数可以使用python中的字典进行实现。我们先定义一个初始字典,包括四个统计项分别对应的键值对。接着,我们遍历输入的字符串,并在遍历过程中利用字符串的isupper()、islower()、isdigit()方法,判断当前字符属于哪种类型,然后将这个类型的值在字典中相应的统计项加1。最后,函数返回更新后的字典即可。
相关问题
python写代码 编写函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以字典的形式返回结果
def count_chars(s):
"""
统计字符串中的大写字母、小写字母、数字、其他字符个数
:param s: 字符串
:return: 字典,包含各类字符个数
"""
count_dict = {'upper': 0, 'lower': 0, 'digit': 0, 'other': 0}
for char in s:
if char.isupper():
count_dict['upper'] += 1
elif char.islower():
count_dict['lower'] += 1
elif char.isdigit():
count_dict['digit'] += 1
else:
count_dict['other'] += 1
return count_dict
python编写函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以字典的形式返回结果。
以下是Python代码实现:
```python
def count_chars(s):
result = {
'uppercase': 0,
'lowercase': 0,
'digit': 0,
'other': 0
} # 初始化结果字典
for c in s:
if c.isupper():
result['uppercase'] += 1
elif c.islower():
result['lowercase'] += 1
elif c.isdigit():
result['digit'] += 1
else:
result['other'] += 1
return result
```
使用示例:
```python
>>> s = 'Hello, World! 123'
>>> count_chars(s)
{'uppercase': 2, 'lowercase': 8, 'digit': 3, 'other': 4}
```
阅读全文
相关推荐
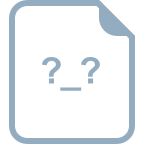
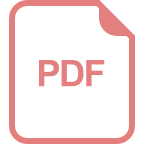












